mirror of
https://github.com/ipfs/kubo.git
synced 2025-07-03 21:08:17 +08:00
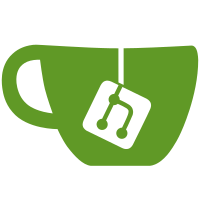
For the rest of the packages in util, move them to thirdparty and update the references. util is gone! License: MIT Signed-off-by: Jeromy <jeromyj@gmail.com>
87 lines
2.1 KiB
Go
87 lines
2.1 KiB
Go
package coreunix
|
|
|
|
import (
|
|
"bytes"
|
|
"io/ioutil"
|
|
"testing"
|
|
|
|
ds "github.com/ipfs/go-ipfs/Godeps/_workspace/src/github.com/ipfs/go-datastore"
|
|
dssync "github.com/ipfs/go-ipfs/Godeps/_workspace/src/github.com/ipfs/go-datastore/sync"
|
|
context "gx/ipfs/QmZy2y8t9zQH2a1b8q2ZSLKp17ATuJoCNxxyMFG5qFExpt/go-net/context"
|
|
|
|
bstore "github.com/ipfs/go-ipfs/blocks/blockstore"
|
|
key "github.com/ipfs/go-ipfs/blocks/key"
|
|
bserv "github.com/ipfs/go-ipfs/blockservice"
|
|
core "github.com/ipfs/go-ipfs/core"
|
|
offline "github.com/ipfs/go-ipfs/exchange/offline"
|
|
importer "github.com/ipfs/go-ipfs/importer"
|
|
chunk "github.com/ipfs/go-ipfs/importer/chunk"
|
|
merkledag "github.com/ipfs/go-ipfs/merkledag"
|
|
ft "github.com/ipfs/go-ipfs/unixfs"
|
|
uio "github.com/ipfs/go-ipfs/unixfs/io"
|
|
u "gx/ipfs/QmZNVWh8LLjAavuQ2JXuFmuYH3C11xo988vSgp7UQrTRj1/go-ipfs-util"
|
|
)
|
|
|
|
func getDagserv(t *testing.T) merkledag.DAGService {
|
|
db := dssync.MutexWrap(ds.NewMapDatastore())
|
|
bs := bstore.NewBlockstore(db)
|
|
blockserv := bserv.New(bs, offline.Exchange(bs))
|
|
return merkledag.NewDAGService(blockserv)
|
|
}
|
|
|
|
func TestMetadata(t *testing.T) {
|
|
ctx := context.Background()
|
|
// Make some random node
|
|
ds := getDagserv(t)
|
|
data := make([]byte, 1000)
|
|
u.NewTimeSeededRand().Read(data)
|
|
r := bytes.NewReader(data)
|
|
nd, err := importer.BuildDagFromReader(ds, chunk.DefaultSplitter(r))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
k, err := nd.Key()
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
m := new(ft.Metadata)
|
|
m.MimeType = "THIS IS A TEST"
|
|
|
|
// Such effort, many compromise
|
|
ipfsnode := &core.IpfsNode{DAG: ds}
|
|
|
|
mdk, err := AddMetadataTo(ipfsnode, k.B58String(), m)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
rec, err := Metadata(ipfsnode, mdk)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if rec.MimeType != m.MimeType {
|
|
t.Fatalf("something went wrong in conversion: '%s' != '%s'", rec.MimeType, m.MimeType)
|
|
}
|
|
|
|
retnode, err := ds.Get(ctx, key.B58KeyDecode(mdk))
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
ndr, err := uio.NewDagReader(ctx, retnode, ds)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
out, err := ioutil.ReadAll(ndr)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
if !bytes.Equal(out, data) {
|
|
t.Fatal("read incorrect data")
|
|
}
|
|
}
|