mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-21 17:08:13 +08:00
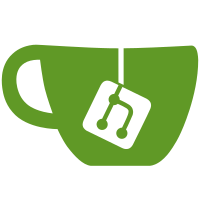
With verbose flag: * remove EnableStdin() flags on all StringArg, * remove all unneeded parsing code for StringArg, and print an * informative message if `ipfs` begins reading from a CharDevice, * remove broken go tests for EnableStdin cli parsing, and add some * trivial test cases for reading FileArg from stdin, * add a panic to prevent EnableStdin from being set on * StringArg in the future. Resolves: #2877, #2870 License: MIT Signed-off-by: Thomas Gardner <tmg@fastmail.com>
80 lines
2.2 KiB
Go
80 lines
2.2 KiB
Go
package commands
|
|
|
|
import (
|
|
"io"
|
|
"strings"
|
|
|
|
cmds "github.com/ipfs/go-ipfs/commands"
|
|
namesys "github.com/ipfs/go-ipfs/namesys"
|
|
util "gx/ipfs/QmZNVWh8LLjAavuQ2JXuFmuYH3C11xo988vSgp7UQrTRj1/go-ipfs-util"
|
|
)
|
|
|
|
var DNSCmd = &cmds.Command{
|
|
Helptext: cmds.HelpText{
|
|
Tagline: "DNS link resolver.",
|
|
ShortDescription: `
|
|
Multihashes are hard to remember, but domain names are usually easy to
|
|
remember. To create memorable aliases for multihashes, DNS TXT
|
|
records can point to other DNS links, IPFS objects, IPNS keys, etc.
|
|
This command resolves those links to the referenced object.
|
|
`,
|
|
LongDescription: `
|
|
Multihashes are hard to remember, but domain names are usually easy to
|
|
remember. To create memorable aliases for multihashes, DNS TXT
|
|
records can point to other DNS links, IPFS objects, IPNS keys, etc.
|
|
This command resolves those links to the referenced object.
|
|
|
|
For example, with this DNS TXT record:
|
|
|
|
> dig +short TXT ipfs.io
|
|
dnslink=/ipfs/QmRzTuh2Lpuz7Gr39stNr6mTFdqAghsZec1JoUnfySUzcy
|
|
|
|
The resolver will give:
|
|
|
|
> ipfs dns ipfs.io
|
|
/ipfs/QmRzTuh2Lpuz7Gr39stNr6mTFdqAghsZec1JoUnfySUzcy
|
|
|
|
The resolver can recursively resolve:
|
|
|
|
> dig +short TXT recursive.ipfs.io
|
|
dnslink=/ipns/ipfs.io
|
|
> ipfs dns -r recursive.ipfs.io
|
|
/ipfs/QmRzTuh2Lpuz7Gr39stNr6mTFdqAghsZec1JoUnfySUzcy
|
|
`,
|
|
},
|
|
|
|
Arguments: []cmds.Argument{
|
|
cmds.StringArg("domain-name", true, false, "The domain-name name to resolve."),
|
|
},
|
|
Options: []cmds.Option{
|
|
cmds.BoolOption("recursive", "r", "Resolve until the result is not a DNS link.").Default(false),
|
|
},
|
|
Run: func(req cmds.Request, res cmds.Response) {
|
|
|
|
recursive, _, _ := req.Option("recursive").Bool()
|
|
name := req.Arguments()[0]
|
|
resolver := namesys.NewDNSResolver()
|
|
|
|
depth := 1
|
|
if recursive {
|
|
depth = namesys.DefaultDepthLimit
|
|
}
|
|
output, err := resolver.ResolveN(req.Context(), name, depth)
|
|
if err != nil {
|
|
res.SetError(err, cmds.ErrNormal)
|
|
return
|
|
}
|
|
res.SetOutput(&ResolvedPath{output})
|
|
},
|
|
Marshalers: cmds.MarshalerMap{
|
|
cmds.Text: func(res cmds.Response) (io.Reader, error) {
|
|
output, ok := res.Output().(*ResolvedPath)
|
|
if !ok {
|
|
return nil, util.ErrCast()
|
|
}
|
|
return strings.NewReader(output.Path.String() + "\n"), nil
|
|
},
|
|
},
|
|
Type: ResolvedPath{},
|
|
}
|