mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-17 23:16:11 +08:00
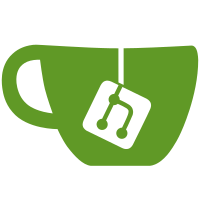
* feat: add block profiling to collect-profiles.sh * feat: add more profiles to 'ipfs diag profile' This adds mutex and block profiles, and brings the command up-to-par with 'collect-profiles.sh', so that we can remove it. Profiles are also now collected concurrently, which improves the runtime from (profile_time * num_profiles) to just (profile_time). Note that this has a backwards-incompatible change, removing --cpu-profile-time in favor of the more general --profile-time, which covers all sampling profiles. * docs(cli): ipfs diag profile * add CLI flag to select specific diag collectors Co-authored-by: Marcin Rataj <lidel@lidel.org>
133 lines
3.5 KiB
Go
133 lines
3.5 KiB
Go
package commands
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"io"
|
|
"runtime/debug"
|
|
|
|
version "github.com/ipfs/go-ipfs"
|
|
|
|
cmds "github.com/ipfs/go-ipfs-cmds"
|
|
)
|
|
|
|
const (
|
|
versionNumberOptionName = "number"
|
|
versionCommitOptionName = "commit"
|
|
versionRepoOptionName = "repo"
|
|
versionAllOptionName = "all"
|
|
)
|
|
|
|
var VersionCmd = &cmds.Command{
|
|
Helptext: cmds.HelpText{
|
|
Tagline: "Show IPFS version information.",
|
|
ShortDescription: "Returns the current version of IPFS and exits.",
|
|
},
|
|
Subcommands: map[string]*cmds.Command{
|
|
"deps": depsVersionCommand,
|
|
},
|
|
|
|
Options: []cmds.Option{
|
|
cmds.BoolOption(versionNumberOptionName, "n", "Only show the version number."),
|
|
cmds.BoolOption(versionCommitOptionName, "Show the commit hash."),
|
|
cmds.BoolOption(versionRepoOptionName, "Show repo version."),
|
|
cmds.BoolOption(versionAllOptionName, "Show all version information"),
|
|
},
|
|
// must be permitted to run before init
|
|
Extra: CreateCmdExtras(SetDoesNotUseRepo(true), SetDoesNotUseConfigAsInput(true)),
|
|
Run: func(req *cmds.Request, res cmds.ResponseEmitter, env cmds.Environment) error {
|
|
return cmds.EmitOnce(res, version.GetVersionInfo())
|
|
},
|
|
Encoders: cmds.EncoderMap{
|
|
cmds.Text: cmds.MakeTypedEncoder(func(req *cmds.Request, w io.Writer, version *version.VersionInfo) error {
|
|
all, _ := req.Options[versionAllOptionName].(bool)
|
|
if all {
|
|
ver := version.Version
|
|
if version.Commit != "" {
|
|
ver += "-" + version.Commit
|
|
}
|
|
out := fmt.Sprintf("go-ipfs version: %s\n"+
|
|
"Repo version: %s\nSystem version: %s\nGolang version: %s\n",
|
|
ver, version.Repo, version.System, version.Golang)
|
|
fmt.Fprint(w, out)
|
|
return nil
|
|
}
|
|
|
|
commit, _ := req.Options[versionCommitOptionName].(bool)
|
|
commitTxt := ""
|
|
if commit && version.Commit != "" {
|
|
commitTxt = "-" + version.Commit
|
|
}
|
|
|
|
repo, _ := req.Options[versionRepoOptionName].(bool)
|
|
if repo {
|
|
fmt.Fprintln(w, version.Repo)
|
|
return nil
|
|
}
|
|
|
|
number, _ := req.Options[versionNumberOptionName].(bool)
|
|
if number {
|
|
fmt.Fprintln(w, version.Version+commitTxt)
|
|
return nil
|
|
}
|
|
|
|
fmt.Fprint(w, fmt.Sprintf("ipfs version %s%s\n", version.Version, commitTxt))
|
|
return nil
|
|
}),
|
|
},
|
|
Type: version.VersionInfo{},
|
|
}
|
|
|
|
type Dependency struct {
|
|
Path string
|
|
Version string
|
|
ReplacedBy string
|
|
Sum string
|
|
}
|
|
|
|
const pkgVersionFmt = "%s@%s"
|
|
|
|
var depsVersionCommand = &cmds.Command{
|
|
Helptext: cmds.HelpText{
|
|
Tagline: "Shows information about dependencies used for build.",
|
|
ShortDescription: `
|
|
Print out all dependencies and their versions.`,
|
|
},
|
|
Type: Dependency{},
|
|
|
|
Run: func(req *cmds.Request, res cmds.ResponseEmitter, env cmds.Environment) error {
|
|
info, ok := debug.ReadBuildInfo()
|
|
if !ok {
|
|
return errors.New("no embedded dependency information")
|
|
}
|
|
toDependency := func(mod *debug.Module) (dep Dependency) {
|
|
dep.Path = mod.Path
|
|
dep.Version = mod.Version
|
|
dep.Sum = mod.Sum
|
|
if repl := mod.Replace; repl != nil {
|
|
dep.ReplacedBy = fmt.Sprintf(pkgVersionFmt, repl.Path, repl.Version)
|
|
}
|
|
return
|
|
}
|
|
if err := res.Emit(toDependency(&info.Main)); err != nil {
|
|
return err
|
|
}
|
|
for _, dep := range info.Deps {
|
|
if err := res.Emit(toDependency(dep)); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
},
|
|
Encoders: cmds.EncoderMap{
|
|
cmds.Text: cmds.MakeTypedEncoder(func(req *cmds.Request, w io.Writer, dep Dependency) error {
|
|
fmt.Fprintf(w, pkgVersionFmt, dep.Path, dep.Version)
|
|
if dep.ReplacedBy != "" {
|
|
fmt.Fprintf(w, " => %s", dep.ReplacedBy)
|
|
}
|
|
fmt.Fprintf(w, "\n")
|
|
return nil
|
|
}),
|
|
},
|
|
}
|