mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-21 00:47:22 +08:00
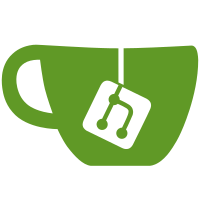
This commit upgrades go-ipfs-cmds and configures the commands HTTP API Handler to only allow POST/OPTIONS, disallowing GET and others in the handling of command requests in the IPFS HTTP API (where before every type of request method was handled, with GET/POST/PUT/PATCH being equivalent). The Read-Only commands that the HTTP API attaches to the gateway endpoint will additional handled GET as they did before (but stop handling PUT,DELETEs). By limiting the request types we address the possibility that a website accessed by a browser abuses the IPFS API by issuing GET requests to it which have no Origin or Referrer set, and are thus bypass CORS and CSRF protections. This is a breaking change for clients that relay on GET requests against the HTTP endpoint (usually :5001). Applications integrating on top of the gateway-read-only API should still work (including cross-domain access). Co-Authored-By: Steven Allen <steven@stebalien.com> Co-Authored-By: Marcin Rataj <lidel@lidel.org>
188 lines
5.5 KiB
Go
188 lines
5.5 KiB
Go
package corehttp
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"net"
|
|
"net/http"
|
|
"os"
|
|
"strconv"
|
|
"strings"
|
|
|
|
version "github.com/ipfs/go-ipfs"
|
|
oldcmds "github.com/ipfs/go-ipfs/commands"
|
|
"github.com/ipfs/go-ipfs/core"
|
|
corecommands "github.com/ipfs/go-ipfs/core/commands"
|
|
|
|
cmds "github.com/ipfs/go-ipfs-cmds"
|
|
cmdsHttp "github.com/ipfs/go-ipfs-cmds/http"
|
|
config "github.com/ipfs/go-ipfs-config"
|
|
path "github.com/ipfs/go-path"
|
|
)
|
|
|
|
var (
|
|
errAPIVersionMismatch = errors.New("api version mismatch")
|
|
)
|
|
|
|
const originEnvKey = "API_ORIGIN"
|
|
const originEnvKeyDeprecate = `You are using the ` + originEnvKey + `ENV Variable.
|
|
This functionality is deprecated, and will be removed in future versions.
|
|
Instead, try either adding headers to the config, or passing them via
|
|
cli arguments:
|
|
|
|
ipfs config API.HTTPHeaders --json '{"Access-Control-Allow-Origin": ["*"]}'
|
|
ipfs daemon
|
|
`
|
|
|
|
// APIPath is the path at which the API is mounted.
|
|
const APIPath = "/api/v0"
|
|
|
|
var defaultLocalhostOrigins = []string{
|
|
"http://127.0.0.1:<port>",
|
|
"https://127.0.0.1:<port>",
|
|
"http://localhost:<port>",
|
|
"https://localhost:<port>",
|
|
}
|
|
|
|
func addCORSFromEnv(c *cmdsHttp.ServerConfig) {
|
|
origin := os.Getenv(originEnvKey)
|
|
if origin != "" {
|
|
log.Warn(originEnvKeyDeprecate)
|
|
c.AppendAllowedOrigins(origin)
|
|
}
|
|
}
|
|
|
|
func addHeadersFromConfig(c *cmdsHttp.ServerConfig, nc *config.Config) {
|
|
log.Info("Using API.HTTPHeaders:", nc.API.HTTPHeaders)
|
|
|
|
if acao := nc.API.HTTPHeaders[cmdsHttp.ACAOrigin]; acao != nil {
|
|
c.SetAllowedOrigins(acao...)
|
|
}
|
|
if acam := nc.API.HTTPHeaders[cmdsHttp.ACAMethods]; acam != nil {
|
|
c.SetAllowedMethods(acam...)
|
|
}
|
|
for _, v := range nc.API.HTTPHeaders[cmdsHttp.ACACredentials] {
|
|
c.SetAllowCredentials(strings.ToLower(v) == "true")
|
|
}
|
|
|
|
c.Headers = make(map[string][]string, len(nc.API.HTTPHeaders)+1)
|
|
|
|
// Copy these because the config is shared and this function is called
|
|
// in multiple places concurrently. Updating these in-place *is* racy.
|
|
for h, v := range nc.API.HTTPHeaders {
|
|
h = http.CanonicalHeaderKey(h)
|
|
switch h {
|
|
case cmdsHttp.ACAOrigin, cmdsHttp.ACAMethods, cmdsHttp.ACACredentials:
|
|
// these are handled by the CORs library.
|
|
default:
|
|
c.Headers[h] = v
|
|
}
|
|
}
|
|
c.Headers["Server"] = []string{"go-ipfs/" + version.CurrentVersionNumber}
|
|
}
|
|
|
|
func addCORSDefaults(c *cmdsHttp.ServerConfig) {
|
|
// by default use localhost origins
|
|
if len(c.AllowedOrigins()) == 0 {
|
|
c.SetAllowedOrigins(defaultLocalhostOrigins...)
|
|
}
|
|
|
|
// by default, use GET, PUT, POST
|
|
if len(c.AllowedMethods()) == 0 {
|
|
c.SetAllowedMethods(http.MethodGet, http.MethodPost, http.MethodPut)
|
|
}
|
|
}
|
|
|
|
func patchCORSVars(c *cmdsHttp.ServerConfig, addr net.Addr) {
|
|
|
|
// we have to grab the port from an addr, which may be an ip6 addr.
|
|
// TODO: this should take multiaddrs and derive port from there.
|
|
port := ""
|
|
if tcpaddr, ok := addr.(*net.TCPAddr); ok {
|
|
port = strconv.Itoa(tcpaddr.Port)
|
|
} else if udpaddr, ok := addr.(*net.UDPAddr); ok {
|
|
port = strconv.Itoa(udpaddr.Port)
|
|
}
|
|
|
|
// we're listening on tcp/udp with ports. ("udp!?" you say? yeah... it happens...)
|
|
oldOrigins := c.AllowedOrigins()
|
|
newOrigins := make([]string, len(oldOrigins))
|
|
for i, o := range oldOrigins {
|
|
// TODO: allow replacing <host>. tricky, ip4 and ip6 and hostnames...
|
|
if port != "" {
|
|
o = strings.Replace(o, "<port>", port, -1)
|
|
}
|
|
newOrigins[i] = o
|
|
}
|
|
c.SetAllowedOrigins(newOrigins...)
|
|
}
|
|
|
|
func commandsOption(cctx oldcmds.Context, command *cmds.Command, allowGet bool) ServeOption {
|
|
return func(n *core.IpfsNode, l net.Listener, mux *http.ServeMux) (*http.ServeMux, error) {
|
|
|
|
cfg := cmdsHttp.NewServerConfig()
|
|
cfg.AllowGet = allowGet
|
|
corsAllowedMethods := []string{http.MethodPost}
|
|
if allowGet {
|
|
corsAllowedMethods = append(corsAllowedMethods, http.MethodGet)
|
|
}
|
|
|
|
cfg.SetAllowedMethods(corsAllowedMethods...)
|
|
cfg.APIPath = APIPath
|
|
rcfg, err := n.Repo.Config()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
addHeadersFromConfig(cfg, rcfg)
|
|
addCORSFromEnv(cfg)
|
|
addCORSDefaults(cfg)
|
|
patchCORSVars(cfg, l.Addr())
|
|
|
|
cmdHandler := cmdsHttp.NewHandler(&cctx, command, cfg)
|
|
mux.Handle(APIPath+"/", cmdHandler)
|
|
return mux, nil
|
|
}
|
|
}
|
|
|
|
// CommandsOption constructs a ServerOption for hooking the commands into the
|
|
// HTTP server. It will NOT allow GET requests.
|
|
func CommandsOption(cctx oldcmds.Context) ServeOption {
|
|
return commandsOption(cctx, corecommands.Root, false)
|
|
}
|
|
|
|
// CommandsROOption constructs a ServerOption for hooking the read-only commands
|
|
// into the HTTP server. It will allow GET requests.
|
|
func CommandsROOption(cctx oldcmds.Context) ServeOption {
|
|
return commandsOption(cctx, corecommands.RootRO, true)
|
|
}
|
|
|
|
// CheckVersionOption returns a ServeOption that checks whether the client ipfs version matches. Does nothing when the user agent string does not contain `/go-ipfs/`
|
|
func CheckVersionOption() ServeOption {
|
|
daemonVersion := version.ApiVersion
|
|
|
|
return ServeOption(func(n *core.IpfsNode, l net.Listener, parent *http.ServeMux) (*http.ServeMux, error) {
|
|
mux := http.NewServeMux()
|
|
parent.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
|
|
if strings.HasPrefix(r.URL.Path, APIPath) {
|
|
cmdqry := r.URL.Path[len(APIPath):]
|
|
pth := path.SplitList(cmdqry)
|
|
|
|
// backwards compatibility to previous version check
|
|
if len(pth) >= 2 && pth[1] != "version" {
|
|
clientVersion := r.UserAgent()
|
|
// skips check if client is not go-ipfs
|
|
if strings.Contains(clientVersion, "/go-ipfs/") && daemonVersion != clientVersion {
|
|
http.Error(w, fmt.Sprintf("%s (%s != %s)", errAPIVersionMismatch, daemonVersion, clientVersion), http.StatusBadRequest)
|
|
return
|
|
}
|
|
}
|
|
}
|
|
|
|
mux.ServeHTTP(w, r)
|
|
})
|
|
|
|
return mux, nil
|
|
})
|
|
}
|