mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-17 15:06:47 +08:00
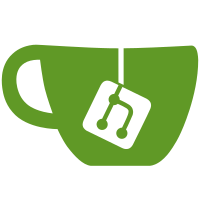
Importantly: * fixes a bunch of MFS bugs * pulls in some bitswap improvements License: MIT Signed-off-by: Steven Allen <steven@stebalien.com>
176 lines
4.9 KiB
Go
176 lines
4.9 KiB
Go
package coreapi
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/ipfs/go-ipfs/core"
|
|
"github.com/ipfs/go-ipfs/filestore"
|
|
|
|
coreiface "github.com/ipfs/go-ipfs/core/coreapi/interface"
|
|
"github.com/ipfs/go-ipfs/core/coreapi/interface/options"
|
|
"github.com/ipfs/go-ipfs/core/coreunix"
|
|
|
|
mfs "gx/ipfs/QmP9eu5X5Ax8169jNWqAJcc42mdZgzLR1aKCEzqhNoBLKk/go-mfs"
|
|
ft "gx/ipfs/QmQXze9tG878pa4Euya4rrDpyTNX3kQe4dhCaBzBozGgpe/go-unixfs"
|
|
uio "gx/ipfs/QmQXze9tG878pa4Euya4rrDpyTNX3kQe4dhCaBzBozGgpe/go-unixfs/io"
|
|
bstore "gx/ipfs/QmS2aqUZLJp8kF1ihE5rvDGE5LvmKDPnx32w9Z1BW9xLV5/go-ipfs-blockstore"
|
|
dag "gx/ipfs/QmTQdH4848iTVCJmKXYyRiK72HufWTLYQQ8iN3JaQ8K1Hq/go-merkledag"
|
|
dagtest "gx/ipfs/QmTQdH4848iTVCJmKXYyRiK72HufWTLYQQ8iN3JaQ8K1Hq/go-merkledag/test"
|
|
files "gx/ipfs/QmXWZCd8jfaHmt4UDSnjKmGcrQMw95bDGWqEeVLVJjoANX/go-ipfs-files"
|
|
blockservice "gx/ipfs/QmYPZzd9VqmJDwxUnThfeSbV1Y5o53aVPDijTB7j7rS9Ep/go-blockservice"
|
|
cidutil "gx/ipfs/QmbfKu17LbMWyGUxHEUns9Wf5Dkm8PT6be4uPhTkk4YvaV/go-cidutil"
|
|
ipld "gx/ipfs/QmcKKBwfz6FyQdHR2jsXrrF6XeSBXYL86anmWNewpFpoF5/go-ipld-format"
|
|
)
|
|
|
|
type UnixfsAPI CoreAPI
|
|
|
|
// Add builds a merkledag node from a reader, adds it to the blockstore,
|
|
// and returns the key representing that node.
|
|
func (api *UnixfsAPI) Add(ctx context.Context, files files.Node, opts ...options.UnixfsAddOption) (coreiface.ResolvedPath, error) {
|
|
settings, prefix, err := options.UnixfsAddOptions(opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
cfg, err := api.repo.Config()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// check if repo will exceed storage limit if added
|
|
// TODO: this doesn't handle the case if the hashed file is already in blocks (deduplicated)
|
|
// TODO: conditional GC is disabled due to it is somehow not possible to pass the size to the daemon
|
|
//if err := corerepo.ConditionalGC(req.Context(), n, uint64(size)); err != nil {
|
|
// res.SetError(err, cmdkit.ErrNormal)
|
|
// return
|
|
//}
|
|
|
|
if settings.NoCopy && !cfg.Experimental.FilestoreEnabled {
|
|
return nil, filestore.ErrFilestoreNotEnabled
|
|
}
|
|
|
|
addblockstore := api.blockstore
|
|
if !(settings.FsCache || settings.NoCopy) {
|
|
addblockstore = bstore.NewGCBlockstore(api.baseBlocks, api.blockstore)
|
|
}
|
|
exch := api.exchange
|
|
pinning := api.pinning
|
|
|
|
if settings.OnlyHash {
|
|
nilnode, err := core.NewNode(ctx, &core.BuildCfg{
|
|
//TODO: need this to be true or all files
|
|
// hashed will be stored in memory!
|
|
NilRepo: true,
|
|
})
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
addblockstore = nilnode.Blockstore
|
|
exch = nilnode.Exchange
|
|
pinning = nilnode.Pinning
|
|
}
|
|
|
|
bserv := blockservice.New(addblockstore, exch) // hash security 001
|
|
dserv := dag.NewDAGService(bserv)
|
|
|
|
fileAdder, err := coreunix.NewAdder(ctx, pinning, addblockstore, dserv)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
fileAdder.Chunker = settings.Chunker
|
|
if settings.Events != nil {
|
|
fileAdder.Out = settings.Events
|
|
fileAdder.Progress = settings.Progress
|
|
}
|
|
fileAdder.Hidden = settings.Hidden
|
|
fileAdder.Wrap = settings.Wrap
|
|
fileAdder.Pin = settings.Pin && !settings.OnlyHash
|
|
fileAdder.Silent = settings.Silent
|
|
fileAdder.RawLeaves = settings.RawLeaves
|
|
fileAdder.NoCopy = settings.NoCopy
|
|
fileAdder.Name = settings.StdinName
|
|
fileAdder.CidBuilder = prefix
|
|
|
|
switch settings.Layout {
|
|
case options.BalancedLayout:
|
|
// Default
|
|
case options.TrickleLayout:
|
|
fileAdder.Trickle = true
|
|
default:
|
|
return nil, fmt.Errorf("unknown layout: %d", settings.Layout)
|
|
}
|
|
|
|
if settings.Inline {
|
|
fileAdder.CidBuilder = cidutil.InlineBuilder{
|
|
Builder: fileAdder.CidBuilder,
|
|
Limit: settings.InlineLimit,
|
|
}
|
|
}
|
|
|
|
if settings.OnlyHash {
|
|
md := dagtest.Mock()
|
|
emptyDirNode := ft.EmptyDirNode()
|
|
// Use the same prefix for the "empty" MFS root as for the file adder.
|
|
emptyDirNode.SetCidBuilder(fileAdder.CidBuilder)
|
|
mr, err := mfs.NewRoot(ctx, md, emptyDirNode, nil)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
fileAdder.SetMfsRoot(mr)
|
|
}
|
|
|
|
nd, err := fileAdder.AddAllAndPin(files)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return coreiface.IpfsPath(nd.Cid()), nil
|
|
}
|
|
|
|
func (api *UnixfsAPI) Get(ctx context.Context, p coreiface.Path) (files.Node, error) {
|
|
ses := api.core().getSession(ctx)
|
|
|
|
nd, err := ses.ResolveNode(ctx, p)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
return newUnixfsFile(ctx, ses.dag, nd)
|
|
}
|
|
|
|
// Ls returns the contents of an IPFS or IPNS object(s) at path p, with the format:
|
|
// `<link base58 hash> <link size in bytes> <link name>`
|
|
func (api *UnixfsAPI) Ls(ctx context.Context, p coreiface.Path) ([]*ipld.Link, error) {
|
|
dagnode, err := api.core().ResolveNode(ctx, p)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var ndlinks []*ipld.Link
|
|
dir, err := uio.NewDirectoryFromNode(api.dag, dagnode)
|
|
switch err {
|
|
case nil:
|
|
l, err := dir.Links(ctx)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
ndlinks = l
|
|
case uio.ErrNotADir:
|
|
ndlinks = dagnode.Links()
|
|
default:
|
|
return nil, err
|
|
}
|
|
|
|
links := make([]*ipld.Link, len(ndlinks))
|
|
for i, l := range ndlinks {
|
|
links[i] = &ipld.Link{Name: l.Name, Size: l.Size, Cid: l.Cid}
|
|
}
|
|
return links, nil
|
|
}
|
|
|
|
func (api *UnixfsAPI) core() *CoreAPI {
|
|
return (*CoreAPI)(api)
|
|
}
|