mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-25 02:46:04 +08:00
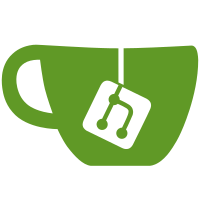
License: MIT Signed-off-by: Brian Tiger Chow <brian@perfmode.com> Conflicts: core/commands/root.go begin ping command, WIP finish initial ping implementation
100 lines
2.4 KiB
Go
100 lines
2.4 KiB
Go
package commands
|
|
|
|
import (
|
|
"io"
|
|
"strings"
|
|
|
|
cmds "github.com/jbenet/go-ipfs/commands"
|
|
u "github.com/jbenet/go-ipfs/util"
|
|
)
|
|
|
|
var log = u.Logger("core/commands")
|
|
|
|
type TestOutput struct {
|
|
Foo string
|
|
Bar int
|
|
}
|
|
|
|
var Root = &cmds.Command{
|
|
Helptext: cmds.HelpText{
|
|
Tagline: "global p2p merkle-dag filesystem",
|
|
Synopsis: `
|
|
ipfs [<flags>] <command> [<arg>] ...
|
|
`,
|
|
ShortDescription: `
|
|
Basic commands:
|
|
|
|
init Initialize ipfs local configuration
|
|
add <path> Add an object to ipfs
|
|
cat <ref> Show ipfs object data
|
|
ls <ref> List links from an object
|
|
|
|
Tool commands:
|
|
|
|
config Manage configuration
|
|
update Download and apply go-ipfs updates
|
|
version Show ipfs version information
|
|
commands List all available commands
|
|
id Show info about ipfs peers
|
|
|
|
Advanced Commands:
|
|
|
|
daemon Start a long-running daemon process
|
|
mount Mount an ipfs read-only mountpoint
|
|
serve Serve an interface to ipfs
|
|
diag Print diagnostics
|
|
|
|
Plumbing commands:
|
|
|
|
block Interact with raw blocks in the datastore
|
|
object Interact with raw dag nodes
|
|
|
|
Use 'ipfs <command> --help' to learn more about each command.
|
|
`,
|
|
},
|
|
Options: []cmds.Option{
|
|
cmds.StringOption("config", "c", "Path to the configuration file to use"),
|
|
cmds.BoolOption("debug", "D", "Operate in debug mode"),
|
|
cmds.BoolOption("help", "Show the full command help text"),
|
|
cmds.BoolOption("h", "Show a short version of the command help text"),
|
|
cmds.BoolOption("local", "L", "Run the command locally, instead of using the daemon"),
|
|
},
|
|
}
|
|
|
|
// commandsDaemonCmd is the "ipfs commands" command for daemon
|
|
var CommandsDaemonCmd = CommandsCmd(Root)
|
|
|
|
var rootSubcommands = map[string]*cmds.Command{
|
|
"add": AddCmd,
|
|
"block": BlockCmd,
|
|
"bootstrap": BootstrapCmd,
|
|
"cat": CatCmd,
|
|
"commands": CommandsDaemonCmd,
|
|
"config": ConfigCmd,
|
|
"diag": DiagCmd,
|
|
"id": IDCmd,
|
|
"log": LogCmd,
|
|
"ls": LsCmd,
|
|
"mount": MountCmd,
|
|
"name": NameCmd,
|
|
"object": ObjectCmd,
|
|
"pin": PinCmd,
|
|
"refs": RefsCmd,
|
|
"swarm": SwarmCmd,
|
|
"ping": PingCmd,
|
|
"update": UpdateCmd,
|
|
"version": VersionCmd,
|
|
}
|
|
|
|
func init() {
|
|
Root.Subcommands = rootSubcommands
|
|
}
|
|
|
|
type MessageOutput struct {
|
|
Message string
|
|
}
|
|
|
|
func MessageTextMarshaler(res cmds.Response) (io.Reader, error) {
|
|
return strings.NewReader(res.Output().(*MessageOutput).Message), nil
|
|
}
|