mirror of
https://github.com/ipfs/kubo.git
synced 2025-07-03 21:08:17 +08:00
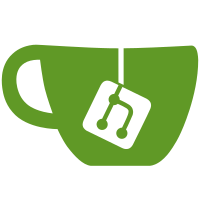
This change adds the /ipfs/bitswap/1.1.0 protocol. The new protocol adds a 'payload' field to the protobuf message and deprecates the existing 'blocks' field. The 'payload' field is an array of pairs of cid prefixes and block data. The cid prefixes are used to ensure the correct codecs and hash functions are used to handle the block on the receiving end. License: MIT Signed-off-by: Jeromy <why@ipfs.io>
57 lines
1.7 KiB
Go
57 lines
1.7 KiB
Go
package proxy
|
|
|
|
import (
|
|
context "context"
|
|
dhtpb "gx/ipfs/QmWHiyk5y2EKgxHogFJ4Zt1xTqKeVsBc4zcBke8ie9C2Bn/go-libp2p-kad-dht/pb"
|
|
ggio "gx/ipfs/QmZ4Qi3GaRbjcx28Sme5eMH7RQjGkt8wHxt2a65oLaeFEV/gogo-protobuf/io"
|
|
inet "gx/ipfs/QmdXimY9QHaasZmw6hWojWnCJvfgxETjZQfg9g6ZrA9wMX/go-libp2p-net"
|
|
peer "gx/ipfs/QmfMmLGoKzCHDN7cGgk64PJr4iipzidDRME8HABSJqvmhC/go-libp2p-peer"
|
|
)
|
|
|
|
// RequestHandler handles routing requests locally
|
|
type RequestHandler interface {
|
|
HandleRequest(ctx context.Context, p peer.ID, m *dhtpb.Message) *dhtpb.Message
|
|
}
|
|
|
|
// Loopback forwards requests to a local handler
|
|
type Loopback struct {
|
|
Handler RequestHandler
|
|
Local peer.ID
|
|
}
|
|
|
|
func (_ *Loopback) Bootstrap(ctx context.Context) error {
|
|
return nil
|
|
}
|
|
|
|
// SendMessage intercepts local requests, forwarding them to a local handler
|
|
func (lb *Loopback) SendMessage(ctx context.Context, m *dhtpb.Message) error {
|
|
response := lb.Handler.HandleRequest(ctx, lb.Local, m)
|
|
if response != nil {
|
|
log.Warning("loopback handler returned unexpected message")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// SendRequest intercepts local requests, forwarding them to a local handler
|
|
func (lb *Loopback) SendRequest(ctx context.Context, m *dhtpb.Message) (*dhtpb.Message, error) {
|
|
return lb.Handler.HandleRequest(ctx, lb.Local, m), nil
|
|
}
|
|
|
|
func (lb *Loopback) HandleStream(s inet.Stream) {
|
|
defer s.Close()
|
|
pbr := ggio.NewDelimitedReader(s, inet.MessageSizeMax)
|
|
var incoming dhtpb.Message
|
|
if err := pbr.ReadMsg(&incoming); err != nil {
|
|
log.Debug(err)
|
|
return
|
|
}
|
|
ctx := context.TODO()
|
|
outgoing := lb.Handler.HandleRequest(ctx, s.Conn().RemotePeer(), &incoming)
|
|
|
|
pbw := ggio.NewDelimitedWriter(s)
|
|
|
|
if err := pbw.WriteMsg(outgoing); err != nil {
|
|
return // TODO logerr
|
|
}
|
|
}
|