mirror of
https://github.com/ipfs/kubo.git
synced 2025-05-20 16:36:46 +08:00
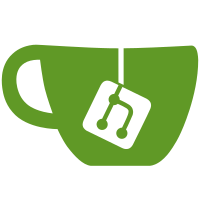
This change adds the /ipfs/bitswap/1.1.0 protocol. The new protocol adds a 'payload' field to the protobuf message and deprecates the existing 'blocks' field. The 'payload' field is an array of pairs of cid prefixes and block data. The cid prefixes are used to ensure the correct codecs and hash functions are used to handle the block on the receiving end. License: MIT Signed-off-by: Jeromy <why@ipfs.io>
111 lines
2.4 KiB
Go
111 lines
2.4 KiB
Go
package dagutils
|
|
|
|
import (
|
|
"testing"
|
|
|
|
dag "github.com/ipfs/go-ipfs/merkledag"
|
|
mdtest "github.com/ipfs/go-ipfs/merkledag/test"
|
|
path "github.com/ipfs/go-ipfs/path"
|
|
|
|
context "context"
|
|
cid "gx/ipfs/QmXUuRadqDq5BuFWzVU6VuKaSjTcNm1gNCtLvvP1TJCW4z/go-cid"
|
|
)
|
|
|
|
func TestAddLink(t *testing.T) {
|
|
ds := mdtest.Mock()
|
|
fishnode := dag.NodeWithData([]byte("fishcakes!"))
|
|
|
|
fk, err := ds.Add(fishnode)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
nd := new(dag.Node)
|
|
nnode, err := addLink(context.Background(), ds, nd, "fish", fishnode)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
fnprime, err := nnode.GetLinkedNode(context.Background(), ds, "fish")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
fnpkey := fnprime.Cid()
|
|
if !fnpkey.Equals(fk) {
|
|
t.Fatal("wrong child node found!")
|
|
}
|
|
}
|
|
|
|
func assertNodeAtPath(t *testing.T, ds dag.DAGService, root *dag.Node, pth string, exp *cid.Cid) {
|
|
parts := path.SplitList(pth)
|
|
cur := root
|
|
for _, e := range parts {
|
|
nxt, err := cur.GetLinkedNode(context.Background(), ds, e)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
cur = nxt
|
|
}
|
|
|
|
curc := cur.Cid()
|
|
if !curc.Equals(exp) {
|
|
t.Fatal("node not as expected at end of path")
|
|
}
|
|
}
|
|
|
|
func TestInsertNode(t *testing.T) {
|
|
root := new(dag.Node)
|
|
e := NewDagEditor(root, nil)
|
|
|
|
testInsert(t, e, "a", "anodefortesting", false, "")
|
|
testInsert(t, e, "a/b", "data", false, "")
|
|
testInsert(t, e, "a/b/c/d/e", "blah", false, "no link by that name")
|
|
testInsert(t, e, "a/b/c/d/e", "foo", true, "")
|
|
testInsert(t, e, "a/b/c/d/f", "baz", true, "")
|
|
testInsert(t, e, "a/b/c/d/f", "bar", true, "")
|
|
|
|
testInsert(t, e, "", "bar", true, "cannot create link with no name!")
|
|
testInsert(t, e, "////", "slashes", true, "cannot create link with no name!")
|
|
|
|
c := e.GetNode().Cid()
|
|
|
|
if c.String() != "QmZ8yeT9uD6ouJPNAYt62XffYuXBT6b4mP4obRSE9cJrSt" {
|
|
t.Fatal("output was different than expected: ", c)
|
|
}
|
|
}
|
|
|
|
func testInsert(t *testing.T, e *Editor, path, data string, create bool, experr string) {
|
|
child := dag.NodeWithData([]byte(data))
|
|
ck, err := e.tmp.Add(child)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
|
|
var c func() *dag.Node
|
|
if create {
|
|
c = func() *dag.Node {
|
|
return &dag.Node{}
|
|
}
|
|
}
|
|
|
|
err = e.InsertNodeAtPath(context.Background(), path, child, c)
|
|
if experr != "" {
|
|
var got string
|
|
if err != nil {
|
|
got = err.Error()
|
|
}
|
|
if got != experr {
|
|
t.Fatalf("expected '%s' but got '%s'", experr, got)
|
|
}
|
|
return
|
|
}
|
|
|
|
if err != nil {
|
|
t.Fatal(err, path, data, create, experr)
|
|
}
|
|
|
|
assertNodeAtPath(t, e.tmp, e.root, path, ck)
|
|
}
|