mirror of
https://github.com/FFmpeg/FFmpeg.git
synced 2025-05-17 15:08:09 +08:00
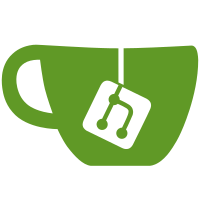
The rounds value is constant and can be one of three hardcoded values, so instead of checking it on every loop, just split the function into three different implementations for each value. Before: aes_decrypt_128_aesni: 93.8 (47.58x) aes_decrypt_192_aesni: 106.9 (49.30x) aes_decrypt_256_aesni: 109.8 (56.50x) aes_encrypt_128_aesni: 93.2 (47.70x) aes_encrypt_192_aesni: 111.1 (48.36x) aes_encrypt_256_aesni: 113.6 (56.27x) After: aes_decrypt_128_aesni: 71.5 (63.31x) aes_decrypt_192_aesni: 96.8 (55.64x) aes_decrypt_256_aesni: 106.1 (58.51x) aes_encrypt_128_aesni: 81.3 (55.92x) aes_encrypt_192_aesni: 91.2 (59.78x) aes_encrypt_256_aesni: 109.0 (58.26x) Signed-off-by: James Almer <jamrial@gmail.com>
51 lines
2.2 KiB
C
51 lines
2.2 KiB
C
/*
|
|
* Copyright (c) 2015 Rodger Combs <rodger.combs@gmail.com>
|
|
*
|
|
* This file is part of FFmpeg.
|
|
*
|
|
* FFmpeg is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU Lesser General Public
|
|
* License as published by the Free Software Foundation; either
|
|
* version 2.1 of the License, or (at your option) any later version.
|
|
*
|
|
* FFmpeg is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
* Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public
|
|
* License along with FFmpeg; if not, write to the Free Software
|
|
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
|
|
*/
|
|
|
|
#include <stddef.h>
|
|
#include "libavutil/aes_internal.h"
|
|
#include "libavutil/x86/cpu.h"
|
|
|
|
void ff_aes_decrypt_10_aesni(AVAES *a, uint8_t *dst, const uint8_t *src,
|
|
int count, uint8_t *iv, int rounds);
|
|
void ff_aes_decrypt_12_aesni(AVAES *a, uint8_t *dst, const uint8_t *src,
|
|
int count, uint8_t *iv, int rounds);
|
|
void ff_aes_decrypt_14_aesni(AVAES *a, uint8_t *dst, const uint8_t *src,
|
|
int count, uint8_t *iv, int rounds);
|
|
void ff_aes_encrypt_10_aesni(AVAES *a, uint8_t *dst, const uint8_t *src,
|
|
int count, uint8_t *iv, int rounds);
|
|
void ff_aes_encrypt_12_aesni(AVAES *a, uint8_t *dst, const uint8_t *src,
|
|
int count, uint8_t *iv, int rounds);
|
|
void ff_aes_encrypt_14_aesni(AVAES *a, uint8_t *dst, const uint8_t *src,
|
|
int count, uint8_t *iv, int rounds);
|
|
|
|
void ff_init_aes_x86(AVAES *a, int decrypt)
|
|
{
|
|
int cpu_flags = av_get_cpu_flags();
|
|
|
|
if (EXTERNAL_AESNI(cpu_flags)) {
|
|
if (a->rounds == 10)
|
|
a->crypt = decrypt ? ff_aes_decrypt_10_aesni : ff_aes_encrypt_10_aesni;
|
|
else if (a->rounds == 12)
|
|
a->crypt = decrypt ? ff_aes_decrypt_12_aesni : ff_aes_encrypt_12_aesni;
|
|
else if (a->rounds == 14)
|
|
a->crypt = decrypt ? ff_aes_decrypt_14_aesni : ff_aes_encrypt_14_aesni;
|
|
}
|
|
}
|