mirror of
https://github.com/rive-app/rive-flutter.git
synced 2025-05-20 06:46:28 +08:00
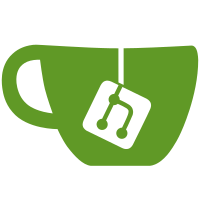
Fixes the problem where FollowPathConstraints were not working with Solos in CPP runtime (works only in editor). The proposed solution is to not collapse constraints of the Solo because in the case of FollowPathConstraint, a call to it's update() is required in order to get the path info. Before the fix: https://github.com/rive-app/rive/assets/186340/48df0eb5-a422-464c-84c0-4c49412fa90e After the fix: https://github.com/rive-app/rive/assets/186340/dbfc8172-217d-4685-92c8-c34c551235ca Diffs= a91b1b764 Don't collapse child constraints of Solos - fixes Follow Paths on Solos (#5866) 033489f8b Fix follow path contention with MetricsPath (#5868) Co-authored-by: Philip Chung <philterdesign@gmail.com>
61 lines
1.5 KiB
Dart
61 lines
1.5 KiB
Dart
import 'package:rive/src/generated/solo_base.dart';
|
|
import 'package:rive/src/rive_core/component.dart';
|
|
import 'package:rive/src/rive_core/constraints/constraint.dart';
|
|
import 'package:rive/src/rive_core/container_component.dart';
|
|
|
|
export 'package:rive/src/generated/solo_base.dart';
|
|
|
|
class Solo extends SoloBase {
|
|
Component? _activeComponent;
|
|
@override
|
|
void activeComponentIdChanged(int from, int to) =>
|
|
activeComponent = context.resolve(to);
|
|
|
|
Component? get activeComponent => _activeComponent;
|
|
|
|
set activeComponent(Component? value) {
|
|
if (_activeComponent == value) {
|
|
return;
|
|
}
|
|
_activeComponent = value;
|
|
activeComponentId = value?.id ?? Core.missingId;
|
|
propagateCollapseToChildren(isCollapsed);
|
|
}
|
|
|
|
@override
|
|
void propagateCollapseToChildren(bool collapse) {
|
|
for (final child in children) {
|
|
if (child is Constraint) {
|
|
continue;
|
|
}
|
|
child.propagateCollapse(collapse || child != _activeComponent);
|
|
}
|
|
}
|
|
|
|
@override
|
|
void childRemoved(Component child) {
|
|
super.childRemoved(child);
|
|
propagateCollapseToChildren(isCollapsed);
|
|
}
|
|
|
|
@override
|
|
void childAdded(Component child) {
|
|
super.childAdded(child);
|
|
propagateCollapseToChildren(isCollapsed);
|
|
}
|
|
|
|
@override
|
|
void onAddedDirty() {
|
|
super.onAddedDirty();
|
|
if (activeComponentId != Core.missingId) {
|
|
activeComponent = context.resolve(activeComponentId);
|
|
}
|
|
}
|
|
|
|
@override
|
|
void onAdded() {
|
|
super.onAdded();
|
|
propagateCollapseToChildren(isCollapsed);
|
|
}
|
|
}
|