mirror of
https://github.com/rive-app/rive-flutter.git
synced 2025-05-17 05:16:05 +08:00
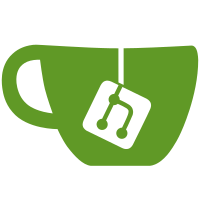
https://github.com/rive-app/rive/assets/186340/00b71832-1293-45fa-b3da-f9abcaa34eb7 Diffs= c8a151ebb Flutter Runtime API for Nested Inputs (#7325) e0a786c90 Runtime API for Nested Inputs (#7316) 01d20e026 Use unique_ptr in import stack. (#7307) 5ad13845d Fail early with bad blend modes. (#7302) Co-authored-by: Philip Chung <philterdesign@gmail.com>
63 lines
2.0 KiB
Dart
63 lines
2.0 KiB
Dart
import 'package:flutter_test/flutter_test.dart';
|
|
import 'package:rive/rive.dart';
|
|
|
|
import 'src/utils.dart';
|
|
|
|
void main() {
|
|
late RiveFile riveFile;
|
|
|
|
setUp(() {
|
|
TestWidgetsFlutterBinding.ensureInitialized();
|
|
final riveBytes = loadFile('assets/runtime_nested_inputs.riv');
|
|
riveFile = RiveFile.import(riveBytes);
|
|
});
|
|
|
|
test('Nested boolean input can be get/set', () async {
|
|
final artboard = riveFile.artboards.first.instance();
|
|
expect(artboard, isNotNull);
|
|
final artboardInstance = artboard.instance();
|
|
expect(artboardInstance, isNotNull);
|
|
final bool = artboard.getBoolInput("CircleOuterState", "CircleOuter");
|
|
expect(bool, isNotNull);
|
|
expect(bool!.value, false);
|
|
bool.value = true;
|
|
expect(bool.value, true);
|
|
});
|
|
|
|
test('Nested number input can be get/set', () async {
|
|
final artboard = riveFile.artboards.first.instance();
|
|
expect(artboard, isNotNull);
|
|
final artboardInstance = artboard.instance();
|
|
expect(artboardInstance, isNotNull);
|
|
final num = artboard.getNumberInput("CircleOuterNumber", "CircleOuter");
|
|
expect(num, isNotNull);
|
|
expect(num!.value, 0);
|
|
num.value = 99;
|
|
expect(num.value, 99);
|
|
});
|
|
|
|
test('Nested trigger can be get/fired', () async {
|
|
final artboard = riveFile.artboards.first.instance();
|
|
expect(artboard, isNotNull);
|
|
final artboardInstance = artboard.instance();
|
|
expect(artboardInstance, isNotNull);
|
|
final trigger =
|
|
artboard.getTriggerInput("CircleOuterTrigger", "CircleOuter");
|
|
expect(trigger, isNotNull);
|
|
trigger!.fire();
|
|
});
|
|
|
|
test('Nested boolean input can be get/set multiple levels deep', () async {
|
|
final artboard = riveFile.artboards.first.instance();
|
|
expect(artboard, isNotNull);
|
|
final artboardInstance = artboard.instance();
|
|
expect(artboardInstance, isNotNull);
|
|
final bool =
|
|
artboard.getBoolInput("CircleInnerState", "CircleOuter/CircleInner");
|
|
expect(bool, isNotNull);
|
|
expect(bool!.value, false);
|
|
bool.value = true;
|
|
expect(bool.value, true);
|
|
});
|
|
}
|