mirror of
https://github.com/rive-app/rive-flutter.git
synced 2025-05-17 05:16:05 +08:00
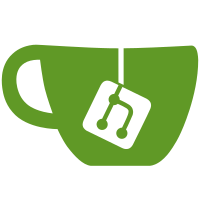
- ran ```dev/generate_core_runtime.sh build``` - cleaned up a lot of accumulated changes from the last few months that needed to be stripped/modified for the rutnime - removed no longer supported lining options from analysis files - fixed up defs for some editor only features so they don't transpile - added some more details to changelog - bumped pubspec version Diffs= aa8c750bd Update flutter runtime (#4835)
69 lines
2.2 KiB
Dart
69 lines
2.2 KiB
Dart
import 'package:flutter_test/flutter_test.dart';
|
|
import 'package:rive/rive.dart';
|
|
|
|
import 'src/utils.dart';
|
|
|
|
void main() {
|
|
late RiveFile riveFile;
|
|
|
|
setUp(() {
|
|
final riveBytes = loadFile('assets/rive-flutter-test-asset.riv');
|
|
riveFile = RiveFile.import(riveBytes);
|
|
});
|
|
|
|
test('LinearAnimationInstance exposes direction is either +/-1', () {
|
|
final artboard = riveFile.mainArtboard;
|
|
|
|
expect(artboard.animations, isNotEmpty);
|
|
LinearAnimationInstance instance =
|
|
LinearAnimationInstance(artboard.animations.first as LinearAnimation);
|
|
|
|
expect(instance.direction, 1);
|
|
instance.direction = -1;
|
|
expect(instance.direction, -1);
|
|
// If any other integer is given, it should default to 1
|
|
instance.direction = -4;
|
|
expect(instance.direction, 1);
|
|
});
|
|
|
|
test('LinearAnimationInstance has a valid start and end time', () {
|
|
final artboard = riveFile.mainArtboard;
|
|
|
|
expect(artboard.animations, isNotEmpty);
|
|
LinearAnimationInstance instance =
|
|
LinearAnimationInstance(artboard.animations.first as LinearAnimation);
|
|
|
|
final startTime = instance.animation.startTime;
|
|
final endTime = instance.animation.endTime;
|
|
expect(startTime <= endTime, isTrue);
|
|
expect(instance.direction, 1);
|
|
// Advance the animation to the mid point
|
|
instance.advance((endTime - startTime) / 2);
|
|
expect(instance.time > startTime, isTrue);
|
|
expect(instance.time < endTime, isTrue);
|
|
// Advance past the end of the4 animation
|
|
instance.advance(endTime);
|
|
expect(instance.time == endTime, isTrue);
|
|
});
|
|
|
|
test('LinearAnimationInstance can be reset', () {
|
|
final artboard = riveFile.mainArtboard;
|
|
|
|
expect(artboard.animations, isNotEmpty);
|
|
LinearAnimationInstance instance =
|
|
LinearAnimationInstance(artboard.animations.first as LinearAnimation);
|
|
|
|
// Check the starting position
|
|
expect(instance.animation.startTime < instance.animation.endTime, isTrue);
|
|
expect(instance.animation.startTime, instance.time);
|
|
|
|
// Advance
|
|
instance.advance(instance.animation.endTime);
|
|
expect(instance.time, instance.animation.endTime);
|
|
|
|
// Reset
|
|
instance.reset();
|
|
expect(instance.time, instance.animation.startTime);
|
|
});
|
|
}
|