mirror of
https://github.com/flutter/packages.git
synced 2025-05-22 11:16:44 +08:00
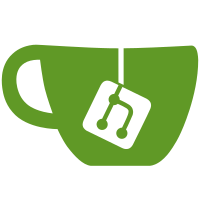
The update-min-sdk command has been using explicitly ranges for the Dart SDK, because that used to be required. Current versions of `pub` no longer require that, and using `^` for Dart ranges is okay, so this updates the tooling to use that format in the future. Also removes the special casing that changed the upper bound from 3 to 4, since we have passed the point where we are generating upper bounds less than 4 anyway. To minimize churn, this doesn't update the existing pubspecs. We can incrementally adopt this going forward as we roll dependencies forward. Fixes https://github.com/flutter/flutter/issues/139806
129 lines
3.9 KiB
Dart
129 lines
3.9 KiB
Dart
// Copyright 2013 The Flutter Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
import 'package:args/command_runner.dart';
|
|
import 'package:file/file.dart';
|
|
import 'package:file/memory.dart';
|
|
import 'package:flutter_plugin_tools/src/update_min_sdk_command.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
import 'util.dart';
|
|
|
|
void main() {
|
|
late FileSystem fileSystem;
|
|
late Directory packagesDir;
|
|
late CommandRunner<void> runner;
|
|
|
|
setUp(() {
|
|
fileSystem = MemoryFileSystem();
|
|
packagesDir = createPackagesDirectory(fileSystem: fileSystem);
|
|
|
|
final UpdateMinSdkCommand command = UpdateMinSdkCommand(
|
|
packagesDir,
|
|
);
|
|
runner = CommandRunner<void>(
|
|
'update_min_sdk_command', 'Test for update_min_sdk_command');
|
|
runner.addCommand(command);
|
|
});
|
|
|
|
test('fails if --flutter-min is missing', () async {
|
|
Error? commandError;
|
|
await runCapturingPrint(runner, <String>[
|
|
'update-min-sdk',
|
|
], errorHandler: (Error e) {
|
|
commandError = e;
|
|
});
|
|
|
|
expect(commandError, isA<ArgumentError>());
|
|
});
|
|
|
|
test('updates Dart when only Dart is present, with manual range', () async {
|
|
final RepositoryPackage package = createFakePackage(
|
|
'a_package', packagesDir,
|
|
dartConstraint: '>=3.0.0 <4.0.0');
|
|
|
|
await runCapturingPrint(runner, <String>[
|
|
'update-min-sdk',
|
|
'--flutter-min',
|
|
'3.13.0', // Corresponds to Dart 3.1.0
|
|
]);
|
|
|
|
final String dartVersion =
|
|
package.parsePubspec().environment?['sdk'].toString() ?? '';
|
|
expect(dartVersion, '^3.1.0');
|
|
});
|
|
|
|
test('updates Dart when only Dart is present, with carrot', () async {
|
|
final RepositoryPackage package =
|
|
createFakePackage('a_package', packagesDir, dartConstraint: '^3.0.0');
|
|
|
|
await runCapturingPrint(runner, <String>[
|
|
'update-min-sdk',
|
|
'--flutter-min',
|
|
'3.13.0', // Corresponds to Dart 3.1.0
|
|
]);
|
|
|
|
final String dartVersion =
|
|
package.parsePubspec().environment?['sdk'].toString() ?? '';
|
|
expect(dartVersion, '^3.1.0');
|
|
});
|
|
|
|
test('does not update Dart if it is already higher', () async {
|
|
final RepositoryPackage package =
|
|
createFakePackage('a_package', packagesDir, dartConstraint: '^3.2.0');
|
|
|
|
await runCapturingPrint(runner, <String>[
|
|
'update-min-sdk',
|
|
'--flutter-min',
|
|
'3.13.0', // Corresponds to Dart 3.1.0
|
|
]);
|
|
|
|
final String dartVersion =
|
|
package.parsePubspec().environment?['sdk'].toString() ?? '';
|
|
expect(dartVersion, '^3.2.0');
|
|
});
|
|
|
|
test('updates both Dart and Flutter when both are present', () async {
|
|
final RepositoryPackage package = createFakePackage(
|
|
'a_package', packagesDir,
|
|
isFlutter: true,
|
|
dartConstraint: '>=3.0.0 <4.0.0',
|
|
flutterConstraint: '>=3.10.0');
|
|
|
|
await runCapturingPrint(runner, <String>[
|
|
'update-min-sdk',
|
|
'--flutter-min',
|
|
'3.13.0', // Corresponds to Dart 3.1.0
|
|
]);
|
|
|
|
final String dartVersion =
|
|
package.parsePubspec().environment?['sdk'].toString() ?? '';
|
|
final String flutterVersion =
|
|
package.parsePubspec().environment?['flutter'].toString() ?? '';
|
|
expect(dartVersion, '^3.1.0');
|
|
expect(flutterVersion, '>=3.13.0');
|
|
});
|
|
|
|
test('does not update Flutter if it is already higher', () async {
|
|
final RepositoryPackage package = createFakePackage(
|
|
'a_package', packagesDir,
|
|
isFlutter: true,
|
|
dartConstraint: '^3.2.0',
|
|
flutterConstraint: '>=3.16.0');
|
|
|
|
await runCapturingPrint(runner, <String>[
|
|
'update-min-sdk',
|
|
'--flutter-min',
|
|
'3.13.0', // Corresponds to Dart 3.1.0
|
|
]);
|
|
|
|
final String dartVersion =
|
|
package.parsePubspec().environment?['sdk'].toString() ?? '';
|
|
final String flutterVersion =
|
|
package.parsePubspec().environment?['flutter'].toString() ?? '';
|
|
expect(dartVersion, '^3.2.0');
|
|
expect(flutterVersion, '>=3.16.0');
|
|
});
|
|
}
|