mirror of
https://github.com/flutter/packages.git
synced 2025-05-22 03:06:49 +08:00
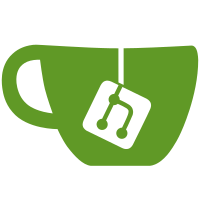
As described in https://github.com/flutter/flutter/issues/121684, we currently have inconsistencies between Flutter SDK constraints and Dart SDK constraints; we have often updated only the former. This PR: 1. Adds CI enforcement via the repo tooling that the minimum versions are consistent. 2. Adds a new repo tooling command to update SDK constraints, to help mass-fix all the violations of the new enforcement in step 1 (and for future mass changes, such as when we update our test matrix and mass-drop support for versions that are no longe tested). - In all cases, the looser constraint was updated to match the more restrictive constraint, such that there's no actual change in what Flutter version any package actually supports. 3. Runs `dart fix --apply` over all changed packages to automatically fix all of the analysis failures caused by step 2 suddenly making all of our packages able to use `super` parameters. Fixes https://github.com/flutter/flutter/issues/121684 Fixes https://github.com/flutter/flutter/issues/121685
103 lines
2.9 KiB
Dart
103 lines
2.9 KiB
Dart
// Copyright 2013 The Flutter Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
import 'package:args/command_runner.dart';
|
|
import 'package:file/file.dart';
|
|
import 'package:file/memory.dart';
|
|
import 'package:flutter_plugin_tools/src/remove_dev_dependencies_command.dart';
|
|
import 'package:test/test.dart';
|
|
|
|
import 'util.dart';
|
|
|
|
void main() {
|
|
late FileSystem fileSystem;
|
|
late Directory packagesDir;
|
|
late CommandRunner<void> runner;
|
|
|
|
setUp(() {
|
|
fileSystem = MemoryFileSystem();
|
|
packagesDir = createPackagesDirectory(fileSystem: fileSystem);
|
|
|
|
final RemoveDevDependenciesCommand command = RemoveDevDependenciesCommand(
|
|
packagesDir,
|
|
);
|
|
runner = CommandRunner<void>('trim_dev_dependencies_command',
|
|
'Test for trim_dev_dependencies_command');
|
|
runner.addCommand(command);
|
|
});
|
|
|
|
void addToPubspec(RepositoryPackage package, String addition) {
|
|
final String originalContent = package.pubspecFile.readAsStringSync();
|
|
package.pubspecFile.writeAsStringSync('''
|
|
$originalContent
|
|
$addition
|
|
''');
|
|
}
|
|
|
|
test('skips if nothing is removed', () async {
|
|
createFakePackage('a_package', packagesDir, version: '1.0.0');
|
|
|
|
final List<String> output =
|
|
await runCapturingPrint(runner, <String>['remove-dev-dependencies']);
|
|
|
|
expect(
|
|
output,
|
|
containsAllInOrder(<Matcher>[
|
|
contains('SKIPPING: Nothing to remove.'),
|
|
]),
|
|
);
|
|
});
|
|
|
|
test('removes dev_dependencies', () async {
|
|
final RepositoryPackage package =
|
|
createFakePackage('a_package', packagesDir, version: '1.0.0');
|
|
|
|
addToPubspec(package, '''
|
|
dev_dependencies:
|
|
some_dependency: ^2.1.8
|
|
another_dependency: ^1.0.0
|
|
''');
|
|
|
|
final List<String> output =
|
|
await runCapturingPrint(runner, <String>['remove-dev-dependencies']);
|
|
|
|
expect(
|
|
output,
|
|
containsAllInOrder(<Matcher>[
|
|
contains('Removed dev_dependencies'),
|
|
]),
|
|
);
|
|
expect(package.pubspecFile.readAsStringSync(),
|
|
isNot(contains('some_dependency:')));
|
|
expect(package.pubspecFile.readAsStringSync(),
|
|
isNot(contains('another_dependency:')));
|
|
});
|
|
|
|
test('removes from examples', () async {
|
|
final RepositoryPackage package =
|
|
createFakePackage('a_package', packagesDir, version: '1.0.0');
|
|
|
|
final RepositoryPackage example = package.getExamples().first;
|
|
addToPubspec(example, '''
|
|
dev_dependencies:
|
|
some_dependency: ^2.1.8
|
|
another_dependency: ^1.0.0
|
|
''');
|
|
|
|
final List<String> output =
|
|
await runCapturingPrint(runner, <String>['remove-dev-dependencies']);
|
|
|
|
expect(
|
|
output,
|
|
containsAllInOrder(<Matcher>[
|
|
contains('Removed dev_dependencies'),
|
|
]),
|
|
);
|
|
expect(package.pubspecFile.readAsStringSync(),
|
|
isNot(contains('some_dependency:')));
|
|
expect(package.pubspecFile.readAsStringSync(),
|
|
isNot(contains('another_dependency:')));
|
|
});
|
|
}
|