mirror of
https://github.com/flutter/packages.git
synced 2025-05-30 04:50:43 +08:00
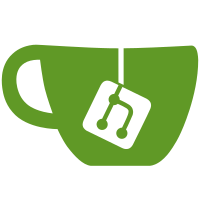
Now that individual commands can be migrated, migrate several commands that are trivially migratable. Part of https://github.com/flutter/flutter/issues/81912
38 lines
1.0 KiB
Dart
38 lines
1.0 KiB
Dart
// Copyright 2013 The Flutter Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style license that can be
|
|
// found in the LICENSE file.
|
|
|
|
import 'dart:async';
|
|
import 'dart:io' as io;
|
|
|
|
import 'package:file/file.dart';
|
|
import 'package:mockito/mockito.dart';
|
|
|
|
class MockProcess extends Mock implements io.Process {
|
|
final Completer<int> exitCodeCompleter = Completer<int>();
|
|
final StreamController<List<int>> stdoutController =
|
|
StreamController<List<int>>();
|
|
final StreamController<List<int>> stderrController =
|
|
StreamController<List<int>>();
|
|
final MockIOSink stdinMock = MockIOSink();
|
|
|
|
@override
|
|
Future<int> get exitCode => exitCodeCompleter.future;
|
|
|
|
@override
|
|
Stream<List<int>> get stdout => stdoutController.stream;
|
|
|
|
@override
|
|
Stream<List<int>> get stderr => stderrController.stream;
|
|
|
|
@override
|
|
IOSink get stdin => stdinMock;
|
|
}
|
|
|
|
class MockIOSink extends Mock implements IOSink {
|
|
List<String> lines = <String>[];
|
|
|
|
@override
|
|
void writeln([Object? obj = '']) => lines.add(obj.toString());
|
|
}
|