mirror of
https://github.com/flutter/holobooth.git
synced 2025-05-17 21:36:00 +08:00
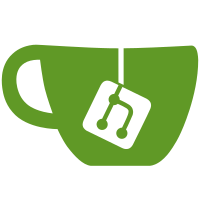
<!-- Thanks for contributing! Provide a description of your changes below and a general summary in the title Please look at the following checklist to ensure that your PR can be accepted quickly: --> ## Description Changes: - Bump min dart SDK to 2.19.0 - Bump Flutter version to 3.7.12 - Used Very Good Analysis 4.0.0 - Corrected new analyzer issues - Dart format ## Type of Change <!--- Put an `x` in all the boxes that apply: --> - [ ] ✨ New feature (non-breaking change which adds functionality) - [ ] 🛠️ Bug fix (non-breaking change which fixes an issue) - [ ] ❌ Breaking change (fix or feature that would cause existing functionality to change) - [ ] 🧹 Code refactor - [ ] ✅ Build configuration change - [ ] 📝 Documentation - [X] 🗑️ Chore
69 lines
1.7 KiB
Dart
69 lines
1.7 KiB
Dart
import 'package:camera/camera.dart';
|
|
import 'package:download_repository/download_repository.dart';
|
|
import 'package:flutter/material.dart';
|
|
import 'package:flutter_bloc/flutter_bloc.dart';
|
|
import 'package:holobooth/convert/convert.dart';
|
|
import 'package:holobooth/footer/footer.dart';
|
|
import 'package:holobooth/share/share.dart';
|
|
import 'package:holobooth_ui/holobooth_ui.dart';
|
|
|
|
class SharePage extends StatelessWidget {
|
|
const SharePage({
|
|
required this.convertBloc,
|
|
this.camera,
|
|
super.key,
|
|
});
|
|
|
|
final ConvertBloc convertBloc;
|
|
final CameraDescription? camera;
|
|
|
|
static Route<void> route({
|
|
required ConvertBloc convertBloc,
|
|
required CameraDescription? camera,
|
|
}) =>
|
|
AppPageRoute(
|
|
builder: (_) => SharePage(
|
|
convertBloc: convertBloc,
|
|
camera: camera,
|
|
),
|
|
);
|
|
|
|
@override
|
|
Widget build(BuildContext context) {
|
|
return MultiBlocProvider(
|
|
providers: [
|
|
BlocProvider.value(value: convertBloc),
|
|
BlocProvider(
|
|
create: (_) => DownloadBloc(
|
|
downloadRepository: context.read<DownloadRepository>(),
|
|
),
|
|
),
|
|
],
|
|
child: Scaffold(body: ShareView(camera: camera)),
|
|
);
|
|
}
|
|
}
|
|
|
|
class ShareView extends StatelessWidget {
|
|
const ShareView({super.key, this.camera});
|
|
|
|
final CameraDescription? camera;
|
|
|
|
@override
|
|
Widget build(BuildContext context) {
|
|
return Stack(
|
|
children: [
|
|
const Positioned.fill(child: ShareBackground()),
|
|
Positioned.fill(
|
|
child: Column(
|
|
children: [
|
|
Expanded(child: ShareBody(camera: camera)),
|
|
FullFooter(footerDecoration: true),
|
|
],
|
|
),
|
|
),
|
|
],
|
|
);
|
|
}
|
|
}
|