mirror of
https://github.com/flutter/holobooth.git
synced 2025-05-17 21:36:00 +08:00
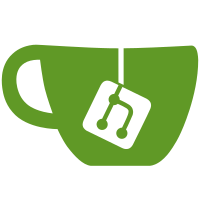
* feat: split characters files * test: coverage * feat: sparky based on platform * test: check by rive asset * chore: format * chore: format * chore: feedback
614 lines
19 KiB
Dart
614 lines
19 KiB
Dart
/// GENERATED CODE - DO NOT MODIFY BY HAND
|
|
/// *****************************************************
|
|
/// FlutterGen
|
|
/// *****************************************************
|
|
|
|
// coverage:ignore-file
|
|
// ignore_for_file: type=lint
|
|
// ignore_for_file: directives_ordering,unnecessary_import,implicit_dynamic_list_literal
|
|
|
|
import 'package:flutter/widgets.dart';
|
|
import 'package:rive/rive.dart';
|
|
|
|
class $AssetsAnimationsGen {
|
|
const $AssetsAnimationsGen();
|
|
|
|
/// File path: assets/animations/bg_00.riv
|
|
RiveGenImage get bg00 => const RiveGenImage('assets/animations/bg_00.riv');
|
|
|
|
/// File path: assets/animations/bg_01.riv
|
|
RiveGenImage get bg01 => const RiveGenImage('assets/animations/bg_01.riv');
|
|
|
|
/// File path: assets/animations/bg_02.riv
|
|
RiveGenImage get bg02 => const RiveGenImage('assets/animations/bg_02.riv');
|
|
|
|
/// File path: assets/animations/bg_03.riv
|
|
RiveGenImage get bg03 => const RiveGenImage('assets/animations/bg_03.riv');
|
|
|
|
/// File path: assets/animations/bg_04.riv
|
|
RiveGenImage get bg04 => const RiveGenImage('assets/animations/bg_04.riv');
|
|
|
|
/// File path: assets/animations/bg_05.riv
|
|
RiveGenImage get bg05 => const RiveGenImage('assets/animations/bg_05.riv');
|
|
|
|
/// File path: assets/animations/bg_06.riv
|
|
RiveGenImage get bg06 => const RiveGenImage('assets/animations/bg_06.riv');
|
|
|
|
/// File path: assets/animations/bg_07.riv
|
|
RiveGenImage get bg07 => const RiveGenImage('assets/animations/bg_07.riv');
|
|
|
|
/// File path: assets/animations/bg_08.riv
|
|
RiveGenImage get bg08 => const RiveGenImage('assets/animations/bg_08.riv');
|
|
|
|
/// File path: assets/animations/dash_desktop.riv
|
|
RiveGenImage get dashDesktop =>
|
|
const RiveGenImage('assets/animations/dash_desktop.riv');
|
|
|
|
/// File path: assets/animations/dash_mobile.riv
|
|
RiveGenImage get dashMobile =>
|
|
const RiveGenImage('assets/animations/dash_mobile.riv');
|
|
|
|
/// File path: assets/animations/sparky_desktop.riv
|
|
RiveGenImage get sparkyDesktop =>
|
|
const RiveGenImage('assets/animations/sparky_desktop.riv');
|
|
|
|
/// File path: assets/animations/sparky_mobile.riv
|
|
RiveGenImage get sparkyMobile =>
|
|
const RiveGenImage('assets/animations/sparky_mobile.riv');
|
|
|
|
/// List of all assets
|
|
List<RiveGenImage> get values => [
|
|
bg00,
|
|
bg01,
|
|
bg02,
|
|
bg03,
|
|
bg04,
|
|
bg05,
|
|
bg06,
|
|
bg07,
|
|
bg08,
|
|
dashDesktop,
|
|
dashMobile,
|
|
sparkyDesktop,
|
|
sparkyMobile
|
|
];
|
|
}
|
|
|
|
class $AssetsAudioGen {
|
|
const $AssetsAudioGen();
|
|
|
|
/// File path: assets/audio/button_press.mp3
|
|
String get buttonPress => 'assets/audio/button_press.mp3';
|
|
|
|
/// File path: assets/audio/counting_3_seconds.mp3
|
|
String get counting3Seconds => 'assets/audio/counting_3_seconds.mp3';
|
|
|
|
/// File path: assets/audio/experience_ambient.mp3
|
|
String get experienceAmbient => 'assets/audio/experience_ambient.mp3';
|
|
|
|
/// File path: assets/audio/face_not_detected.mp3
|
|
String get faceNotDetected => 'assets/audio/face_not_detected.mp3';
|
|
|
|
/// File path: assets/audio/loading.mp3
|
|
String get loading => 'assets/audio/loading.mp3';
|
|
|
|
/// File path: assets/audio/loading_finished.mp3
|
|
String get loadingFinished => 'assets/audio/loading_finished.mp3';
|
|
|
|
/// File path: assets/audio/tab_click.mp3
|
|
String get tabClick => 'assets/audio/tab_click.mp3';
|
|
|
|
/// List of all assets
|
|
List<String> get values => [
|
|
buttonPress,
|
|
counting3Seconds,
|
|
experienceAmbient,
|
|
faceNotDetected,
|
|
loading,
|
|
loadingFinished,
|
|
tabClick
|
|
];
|
|
}
|
|
|
|
class $AssetsBackgroundsGen {
|
|
const $AssetsBackgroundsGen();
|
|
|
|
/// File path: assets/backgrounds/animoji_intro_background.png
|
|
AssetGenImage get animojiIntroBackground =>
|
|
const AssetGenImage('assets/backgrounds/animoji_intro_background.png');
|
|
|
|
/// File path: assets/backgrounds/bg_00.png
|
|
AssetGenImage get bg00 => const AssetGenImage('assets/backgrounds/bg_00.png');
|
|
|
|
/// File path: assets/backgrounds/bg_01.png
|
|
AssetGenImage get bg01 => const AssetGenImage('assets/backgrounds/bg_01.png');
|
|
|
|
/// File path: assets/backgrounds/bg_02.png
|
|
AssetGenImage get bg02 => const AssetGenImage('assets/backgrounds/bg_02.png');
|
|
|
|
/// File path: assets/backgrounds/bg_03.png
|
|
AssetGenImage get bg03 => const AssetGenImage('assets/backgrounds/bg_03.png');
|
|
|
|
/// File path: assets/backgrounds/bg_04.png
|
|
AssetGenImage get bg04 => const AssetGenImage('assets/backgrounds/bg_04.png');
|
|
|
|
/// File path: assets/backgrounds/bg_05.png
|
|
AssetGenImage get bg05 => const AssetGenImage('assets/backgrounds/bg_05.png');
|
|
|
|
/// File path: assets/backgrounds/bg_06.png
|
|
AssetGenImage get bg06 => const AssetGenImage('assets/backgrounds/bg_06.png');
|
|
|
|
/// File path: assets/backgrounds/bg_07.png
|
|
AssetGenImage get bg07 => const AssetGenImage('assets/backgrounds/bg_07.png');
|
|
|
|
/// File path: assets/backgrounds/bg_08.png
|
|
AssetGenImage get bg08 => const AssetGenImage('assets/backgrounds/bg_08.png');
|
|
|
|
/// File path: assets/backgrounds/holobooth.png
|
|
AssetGenImage get holobooth =>
|
|
const AssetGenImage('assets/backgrounds/holobooth.png');
|
|
|
|
/// File path: assets/backgrounds/landing_background.png
|
|
AssetGenImage get landingBackground =>
|
|
const AssetGenImage('assets/backgrounds/landing_background.png');
|
|
|
|
/// File path: assets/backgrounds/loading_background.png
|
|
AssetGenImage get loadingBackground =>
|
|
const AssetGenImage('assets/backgrounds/loading_background.png');
|
|
|
|
/// File path: assets/backgrounds/share_background.png
|
|
AssetGenImage get shareBackground =>
|
|
const AssetGenImage('assets/backgrounds/share_background.png');
|
|
|
|
/// File path: assets/backgrounds/video_frame.png
|
|
AssetGenImage get videoFrame =>
|
|
const AssetGenImage('assets/backgrounds/video_frame.png');
|
|
|
|
/// List of all assets
|
|
List<AssetGenImage> get values => [
|
|
animojiIntroBackground,
|
|
bg00,
|
|
bg01,
|
|
bg02,
|
|
bg03,
|
|
bg04,
|
|
bg05,
|
|
bg06,
|
|
bg07,
|
|
bg08,
|
|
holobooth,
|
|
landingBackground,
|
|
loadingBackground,
|
|
shareBackground,
|
|
videoFrame
|
|
];
|
|
}
|
|
|
|
class $AssetsCharactersGen {
|
|
const $AssetsCharactersGen();
|
|
|
|
/// File path: assets/characters/dash.png
|
|
AssetGenImage get dash => const AssetGenImage('assets/characters/dash.png');
|
|
|
|
/// File path: assets/characters/sparky.png
|
|
AssetGenImage get sparky =>
|
|
const AssetGenImage('assets/characters/sparky.png');
|
|
|
|
/// List of all assets
|
|
List<AssetGenImage> get values => [dash, sparky];
|
|
}
|
|
|
|
class $AssetsIconsGen {
|
|
const $AssetsIconsGen();
|
|
|
|
/// File path: assets/icons/camera_button_icon.png
|
|
AssetGenImage get cameraButtonIcon =>
|
|
const AssetGenImage('assets/icons/camera_button_icon.png');
|
|
|
|
/// File path: assets/icons/classic_photobooth.png
|
|
AssetGenImage get classicPhotobooth =>
|
|
const AssetGenImage('assets/icons/classic_photobooth.png');
|
|
|
|
/// File path: assets/icons/close_icon.png
|
|
AssetGenImage get closeIcon =>
|
|
const AssetGenImage('assets/icons/close_icon.png');
|
|
|
|
/// File path: assets/icons/facebook_logo.png
|
|
AssetGenImage get facebookLogo =>
|
|
const AssetGenImage('assets/icons/facebook_logo.png');
|
|
|
|
/// File path: assets/icons/firebase_icon.png
|
|
AssetGenImage get firebaseIcon =>
|
|
const AssetGenImage('assets/icons/firebase_icon.png');
|
|
|
|
/// File path: assets/icons/flutter_icon.png
|
|
AssetGenImage get flutterIcon =>
|
|
const AssetGenImage('assets/icons/flutter_icon.png');
|
|
|
|
/// File path: assets/icons/go_next_button_icon.png
|
|
AssetGenImage get goNextButtonIcon =>
|
|
const AssetGenImage('assets/icons/go_next_button_icon.png');
|
|
|
|
/// File path: assets/icons/loading circle.png
|
|
AssetGenImage get loadingCircle =>
|
|
const AssetGenImage('assets/icons/loading circle.png');
|
|
|
|
/// File path: assets/icons/loading_finish.png
|
|
AssetGenImage get loadingFinish =>
|
|
const AssetGenImage('assets/icons/loading_finish.png');
|
|
|
|
/// File path: assets/icons/mediapipe_icon.png
|
|
AssetGenImage get mediapipeIcon =>
|
|
const AssetGenImage('assets/icons/mediapipe_icon.png');
|
|
|
|
/// File path: assets/icons/play_icon.png
|
|
AssetGenImage get playIcon =>
|
|
const AssetGenImage('assets/icons/play_icon.png');
|
|
|
|
/// File path: assets/icons/recording_button_icon.png
|
|
AssetGenImage get recordingButtonIcon =>
|
|
const AssetGenImage('assets/icons/recording_button_icon.png');
|
|
|
|
/// File path: assets/icons/retake_button_icon.png
|
|
AssetGenImage get retakeButtonIcon =>
|
|
const AssetGenImage('assets/icons/retake_button_icon.png');
|
|
|
|
/// File path: assets/icons/tensorflow_icon.png
|
|
AssetGenImage get tensorflowIcon =>
|
|
const AssetGenImage('assets/icons/tensorflow_icon.png');
|
|
|
|
/// File path: assets/icons/twitter_logo.png
|
|
AssetGenImage get twitterLogo =>
|
|
const AssetGenImage('assets/icons/twitter_logo.png');
|
|
|
|
/// List of all assets
|
|
List<AssetGenImage> get values => [
|
|
cameraButtonIcon,
|
|
classicPhotobooth,
|
|
closeIcon,
|
|
facebookLogo,
|
|
firebaseIcon,
|
|
flutterIcon,
|
|
goNextButtonIcon,
|
|
loadingCircle,
|
|
loadingFinish,
|
|
mediapipeIcon,
|
|
playIcon,
|
|
recordingButtonIcon,
|
|
retakeButtonIcon,
|
|
tensorflowIcon,
|
|
twitterLogo
|
|
];
|
|
}
|
|
|
|
class $AssetsImagesGen {
|
|
const $AssetsImagesGen();
|
|
|
|
/// File path: assets/images/flutter_forward_logo.png
|
|
AssetGenImage get flutterForwardLogo =>
|
|
const AssetGenImage('assets/images/flutter_forward_logo.png');
|
|
|
|
/// File path: assets/images/holobooth_avatar.png
|
|
AssetGenImage get holoboothAvatar =>
|
|
const AssetGenImage('assets/images/holobooth_avatar.png');
|
|
|
|
/// List of all assets
|
|
List<AssetGenImage> get values => [flutterForwardLogo, holoboothAvatar];
|
|
}
|
|
|
|
class $AssetsPropsGen {
|
|
const $AssetsPropsGen();
|
|
|
|
/// File path: assets/props/clothes.png
|
|
AssetGenImage get clothes => const AssetGenImage('assets/props/clothes.png');
|
|
|
|
/// File path: assets/props/glasses_01.png
|
|
AssetGenImage get glasses01 =>
|
|
const AssetGenImage('assets/props/glasses_01.png');
|
|
|
|
/// File path: assets/props/glasses_02.png
|
|
AssetGenImage get glasses02 =>
|
|
const AssetGenImage('assets/props/glasses_02.png');
|
|
|
|
/// File path: assets/props/glasses_03.png
|
|
AssetGenImage get glasses03 =>
|
|
const AssetGenImage('assets/props/glasses_03.png');
|
|
|
|
/// File path: assets/props/glasses_04.png
|
|
AssetGenImage get glasses04 =>
|
|
const AssetGenImage('assets/props/glasses_04.png');
|
|
|
|
/// File path: assets/props/glasses_05.png
|
|
AssetGenImage get glasses05 =>
|
|
const AssetGenImage('assets/props/glasses_05.png');
|
|
|
|
/// File path: assets/props/glasses_06.png
|
|
AssetGenImage get glasses06 =>
|
|
const AssetGenImage('assets/props/glasses_06.png');
|
|
|
|
/// File path: assets/props/glasses_07.png
|
|
AssetGenImage get glasses07 =>
|
|
const AssetGenImage('assets/props/glasses_07.png');
|
|
|
|
/// File path: assets/props/glasses_08.png
|
|
AssetGenImage get glasses08 =>
|
|
const AssetGenImage('assets/props/glasses_08.png');
|
|
|
|
/// File path: assets/props/glasses_09.png
|
|
AssetGenImage get glasses09 =>
|
|
const AssetGenImage('assets/props/glasses_09.png');
|
|
|
|
/// File path: assets/props/glasses_icon.png
|
|
AssetGenImage get glassesIcon =>
|
|
const AssetGenImage('assets/props/glasses_icon.png');
|
|
|
|
/// File path: assets/props/handheld_01.png
|
|
AssetGenImage get handheld01 =>
|
|
const AssetGenImage('assets/props/handheld_01.png');
|
|
|
|
/// File path: assets/props/handheld_02.png
|
|
AssetGenImage get handheld02 =>
|
|
const AssetGenImage('assets/props/handheld_02.png');
|
|
|
|
/// File path: assets/props/handheld_03.png
|
|
AssetGenImage get handheld03 =>
|
|
const AssetGenImage('assets/props/handheld_03.png');
|
|
|
|
/// File path: assets/props/handheld_04.png
|
|
AssetGenImage get handheld04 =>
|
|
const AssetGenImage('assets/props/handheld_04.png');
|
|
|
|
/// File path: assets/props/handheld_05.png
|
|
AssetGenImage get handheld05 =>
|
|
const AssetGenImage('assets/props/handheld_05.png');
|
|
|
|
/// File path: assets/props/handheld_06.png
|
|
AssetGenImage get handheld06 =>
|
|
const AssetGenImage('assets/props/handheld_06.png');
|
|
|
|
/// File path: assets/props/handheld_07.png
|
|
AssetGenImage get handheld07 =>
|
|
const AssetGenImage('assets/props/handheld_07.png');
|
|
|
|
/// File path: assets/props/handheld_08.png
|
|
AssetGenImage get handheld08 =>
|
|
const AssetGenImage('assets/props/handheld_08.png');
|
|
|
|
/// File path: assets/props/handheld_09.png
|
|
AssetGenImage get handheld09 =>
|
|
const AssetGenImage('assets/props/handheld_09.png');
|
|
|
|
/// File path: assets/props/handheld_10.png
|
|
AssetGenImage get handheld10 =>
|
|
const AssetGenImage('assets/props/handheld_10.png');
|
|
|
|
/// File path: assets/props/handheld_11.png
|
|
AssetGenImage get handheld11 =>
|
|
const AssetGenImage('assets/props/handheld_11.png');
|
|
|
|
/// File path: assets/props/hat_01.png
|
|
AssetGenImage get hat01 => const AssetGenImage('assets/props/hat_01.png');
|
|
|
|
/// File path: assets/props/hat_02.png
|
|
AssetGenImage get hat02 => const AssetGenImage('assets/props/hat_02.png');
|
|
|
|
/// File path: assets/props/hat_03.png
|
|
AssetGenImage get hat03 => const AssetGenImage('assets/props/hat_03.png');
|
|
|
|
/// File path: assets/props/hat_04.png
|
|
AssetGenImage get hat04 => const AssetGenImage('assets/props/hat_04.png');
|
|
|
|
/// File path: assets/props/hat_05.png
|
|
AssetGenImage get hat05 => const AssetGenImage('assets/props/hat_05.png');
|
|
|
|
/// File path: assets/props/hat_06.png
|
|
AssetGenImage get hat06 => const AssetGenImage('assets/props/hat_06.png');
|
|
|
|
/// File path: assets/props/hat_07.png
|
|
AssetGenImage get hat07 => const AssetGenImage('assets/props/hat_07.png');
|
|
|
|
/// File path: assets/props/hat_08.png
|
|
AssetGenImage get hat08 => const AssetGenImage('assets/props/hat_08.png');
|
|
|
|
/// File path: assets/props/hat_09.png
|
|
AssetGenImage get hat09 => const AssetGenImage('assets/props/hat_09.png');
|
|
|
|
/// File path: assets/props/hats_icon.png
|
|
AssetGenImage get hatsIcon =>
|
|
const AssetGenImage('assets/props/hats_icon.png');
|
|
|
|
/// File path: assets/props/none_props.png
|
|
AssetGenImage get noneProps =>
|
|
const AssetGenImage('assets/props/none_props.png');
|
|
|
|
/// File path: assets/props/others_icon.png
|
|
AssetGenImage get othersIcon =>
|
|
const AssetGenImage('assets/props/others_icon.png');
|
|
|
|
/// File path: assets/props/shirt_01.png
|
|
AssetGenImage get shirt01 => const AssetGenImage('assets/props/shirt_01.png');
|
|
|
|
/// File path: assets/props/shirt_02.png
|
|
AssetGenImage get shirt02 => const AssetGenImage('assets/props/shirt_02.png');
|
|
|
|
/// File path: assets/props/shirt_03.png
|
|
AssetGenImage get shirt03 => const AssetGenImage('assets/props/shirt_03.png');
|
|
|
|
/// File path: assets/props/shirt_04.png
|
|
AssetGenImage get shirt04 => const AssetGenImage('assets/props/shirt_04.png');
|
|
|
|
/// File path: assets/props/shirt_05.png
|
|
AssetGenImage get shirt05 => const AssetGenImage('assets/props/shirt_05.png');
|
|
|
|
/// File path: assets/props/shirt_06.png
|
|
AssetGenImage get shirt06 => const AssetGenImage('assets/props/shirt_06.png');
|
|
|
|
/// File path: assets/props/shirt_07.png
|
|
AssetGenImage get shirt07 => const AssetGenImage('assets/props/shirt_07.png');
|
|
|
|
/// File path: assets/props/shirt_08.png
|
|
AssetGenImage get shirt08 => const AssetGenImage('assets/props/shirt_08.png');
|
|
|
|
/// File path: assets/props/shirt_09.png
|
|
AssetGenImage get shirt09 => const AssetGenImage('assets/props/shirt_09.png');
|
|
|
|
/// List of all assets
|
|
List<AssetGenImage> get values => [
|
|
clothes,
|
|
glasses01,
|
|
glasses02,
|
|
glasses03,
|
|
glasses04,
|
|
glasses05,
|
|
glasses06,
|
|
glasses07,
|
|
glasses08,
|
|
glasses09,
|
|
glassesIcon,
|
|
handheld01,
|
|
handheld02,
|
|
handheld03,
|
|
handheld04,
|
|
handheld05,
|
|
handheld06,
|
|
handheld07,
|
|
handheld08,
|
|
handheld09,
|
|
handheld10,
|
|
handheld11,
|
|
hat01,
|
|
hat02,
|
|
hat03,
|
|
hat04,
|
|
hat05,
|
|
hat06,
|
|
hat07,
|
|
hat08,
|
|
hat09,
|
|
hatsIcon,
|
|
noneProps,
|
|
othersIcon,
|
|
shirt01,
|
|
shirt02,
|
|
shirt03,
|
|
shirt04,
|
|
shirt05,
|
|
shirt06,
|
|
shirt07,
|
|
shirt08,
|
|
shirt09
|
|
];
|
|
}
|
|
|
|
class Assets {
|
|
Assets._();
|
|
|
|
static const $AssetsAnimationsGen animations = $AssetsAnimationsGen();
|
|
static const $AssetsAudioGen audio = $AssetsAudioGen();
|
|
static const $AssetsBackgroundsGen backgrounds = $AssetsBackgroundsGen();
|
|
static const $AssetsCharactersGen characters = $AssetsCharactersGen();
|
|
static const $AssetsIconsGen icons = $AssetsIconsGen();
|
|
static const $AssetsImagesGen images = $AssetsImagesGen();
|
|
static const $AssetsPropsGen props = $AssetsPropsGen();
|
|
}
|
|
|
|
class AssetGenImage {
|
|
const AssetGenImage(this._assetName);
|
|
|
|
final String _assetName;
|
|
|
|
Image image({
|
|
Key? key,
|
|
AssetBundle? bundle,
|
|
ImageFrameBuilder? frameBuilder,
|
|
ImageErrorWidgetBuilder? errorBuilder,
|
|
String? semanticLabel,
|
|
bool excludeFromSemantics = false,
|
|
double? scale,
|
|
double? width,
|
|
double? height,
|
|
Color? color,
|
|
Animation<double>? opacity,
|
|
BlendMode? colorBlendMode,
|
|
BoxFit? fit,
|
|
AlignmentGeometry alignment = Alignment.center,
|
|
ImageRepeat repeat = ImageRepeat.noRepeat,
|
|
Rect? centerSlice,
|
|
bool matchTextDirection = false,
|
|
bool gaplessPlayback = false,
|
|
bool isAntiAlias = false,
|
|
String? package,
|
|
FilterQuality filterQuality = FilterQuality.low,
|
|
int? cacheWidth,
|
|
int? cacheHeight,
|
|
}) {
|
|
return Image.asset(
|
|
_assetName,
|
|
key: key,
|
|
bundle: bundle,
|
|
frameBuilder: frameBuilder,
|
|
errorBuilder: errorBuilder,
|
|
semanticLabel: semanticLabel,
|
|
excludeFromSemantics: excludeFromSemantics,
|
|
scale: scale,
|
|
width: width,
|
|
height: height,
|
|
color: color,
|
|
opacity: opacity,
|
|
colorBlendMode: colorBlendMode,
|
|
fit: fit,
|
|
alignment: alignment,
|
|
repeat: repeat,
|
|
centerSlice: centerSlice,
|
|
matchTextDirection: matchTextDirection,
|
|
gaplessPlayback: gaplessPlayback,
|
|
isAntiAlias: isAntiAlias,
|
|
package: package,
|
|
filterQuality: filterQuality,
|
|
cacheWidth: cacheWidth,
|
|
cacheHeight: cacheHeight,
|
|
);
|
|
}
|
|
|
|
ImageProvider provider() => AssetImage(_assetName);
|
|
|
|
String get path => _assetName;
|
|
|
|
String get keyName => _assetName;
|
|
}
|
|
|
|
class RiveGenImage {
|
|
const RiveGenImage(this._assetName);
|
|
|
|
final String _assetName;
|
|
|
|
RiveAnimation rive({
|
|
String? artboard,
|
|
List<String> animations = const [],
|
|
List<String> stateMachines = const [],
|
|
BoxFit? fit,
|
|
Alignment? alignment,
|
|
Widget? placeHolder,
|
|
bool antialiasing = true,
|
|
List<RiveAnimationController> controllers = const [],
|
|
OnInitCallback? onInit,
|
|
}) {
|
|
return RiveAnimation.asset(
|
|
_assetName,
|
|
artboard: artboard,
|
|
animations: animations,
|
|
stateMachines: stateMachines,
|
|
fit: fit,
|
|
alignment: alignment,
|
|
placeHolder: placeHolder,
|
|
antialiasing: antialiasing,
|
|
controllers: controllers,
|
|
onInit: onInit,
|
|
);
|
|
}
|
|
|
|
String get path => _assetName;
|
|
|
|
String get keyName => _assetName;
|
|
}
|