mirror of
https://github.com/mdanics/fluttergram.git
synced 2025-07-02 12:56:22 +08:00
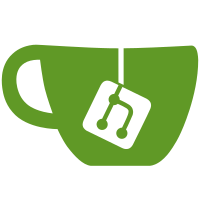
* Real fix for not showing your own posts (#55) Instead of adding yourself as your follower at the moment of the creation of the user, it's better to change the getFeed.ts script to getAllPosts from users that you are following + posts from you. * add same users posts in Feed function (refactor old hack way) Co-authored-by: Gustavo Contreiras <guto.contreiras@gmail.com>
61 lines
2.5 KiB
JavaScript
61 lines
2.5 KiB
JavaScript
"use strict";
|
|
var __awaiter = (this && this.__awaiter) || function (thisArg, _arguments, P, generator) {
|
|
return new (P || (P = Promise))(function (resolve, reject) {
|
|
function fulfilled(value) { try { step(generator.next(value)); } catch (e) { reject(e); } }
|
|
function rejected(value) { try { step(generator["throw"](value)); } catch (e) { reject(e); } }
|
|
function step(result) { result.done ? resolve(result.value) : new P(function (resolve) { resolve(result.value); }).then(fulfilled, rejected); }
|
|
step((generator = generator.apply(thisArg, _arguments || [])).next());
|
|
});
|
|
};
|
|
Object.defineProperty(exports, "__esModule", { value: true });
|
|
const admin = require("firebase-admin");
|
|
exports.getFeedModule = function (req, res) {
|
|
const uid = String(req.query.uid);
|
|
function compileFeedPost() {
|
|
return __awaiter(this, void 0, void 0, function* () {
|
|
const following = yield getFollowing(uid, res);
|
|
let listOfPosts = yield getAllPosts(following, uid, res);
|
|
listOfPosts = [].concat.apply([], listOfPosts); // flattens list
|
|
res.send(listOfPosts);
|
|
});
|
|
}
|
|
compileFeedPost().then().catch();
|
|
};
|
|
function getAllPosts(following, uid, res) {
|
|
return __awaiter(this, void 0, void 0, function* () {
|
|
const listOfPosts = [];
|
|
for (const user in following) {
|
|
listOfPosts.push(yield getUserPosts(following[user], res));
|
|
}
|
|
// add the current user's posts to the feed so that your own posts appear in your feed
|
|
listOfPosts.push(yield getUserPosts(uid, res));
|
|
return listOfPosts;
|
|
});
|
|
}
|
|
function getUserPosts(userId, res) {
|
|
const posts = admin.firestore().collection("insta_posts").where("ownerId", "==", userId).orderBy("timestamp");
|
|
return posts.get()
|
|
.then(function (querySnapshot) {
|
|
const listOfPosts = [];
|
|
querySnapshot.forEach(function (doc) {
|
|
listOfPosts.push(doc.data());
|
|
});
|
|
return listOfPosts;
|
|
});
|
|
}
|
|
function getFollowing(uid, res) {
|
|
const doc = admin.firestore().doc(`insta_users/${uid}`);
|
|
return doc.get().then(snapshot => {
|
|
const followings = snapshot.data().following;
|
|
const following_list = [];
|
|
for (const following in followings) {
|
|
if (followings[following] === true) {
|
|
following_list.push(following);
|
|
}
|
|
}
|
|
return following_list;
|
|
}).catch(error => {
|
|
res.status(500).send(error);
|
|
});
|
|
}
|
|
//# sourceMappingURL=getFeed.js.map
|