mirror of
https://github.com/alibaba/flutter-go.git
synced 2025-05-21 23:06:33 +08:00
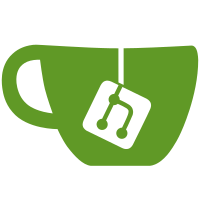
# Conflicts: # lib/widgets/components/Bar/AppBar/index.dart # lib/widgets/components/Bar/BottomAppBar/demo.dart # lib/widgets/components/Bar/BottomAppBar/index.dart # lib/widgets/components/Bar/ButtonBar/index.dart # lib/widgets/components/Bar/FlexibleSpaceBar/index.dart # lib/widgets/components/Bar/SliverAppBar/index.dart # lib/widgets/components/Bar/SnackBar/index.dart # lib/widgets/components/Bar/SnackBarAction/index.dart # lib/widgets/components/Card/Card/index.dart # lib/widgets/components/LIst/ListBody/index.dart # lib/widgets/components/LIst/ListView/index.dart # lib/widgets/elements/Form/Button/DropdownButton/index.dart # lib/widgets/elements/Form/Button/FlatButton/index.dart # lib/widgets/elements/Form/Button/PopupMenuButton/index.dart # lib/widgets/elements/Form/CheckBox/Checkbox/index.dart # lib/widgets/elements/Form/CheckBox/CheckboxListTile/index.dart # lib/widgets/elements/Frame/Axis/crossAxis/index.dart # lib/widgets/elements/Frame/Axis/flipAxis/index.dart # lib/widgets/elements/Frame/Axis/mainAxis/index.dart # lib/widgets/elements/Media/Image/precacheImage/index.dart
69 lines
1.9 KiB
Dart
69 lines
1.9 KiB
Dart
/**
|
||
* Created with Android Studio.
|
||
* User: ryan
|
||
* Date: 2018/12/27
|
||
* Time: 下午6:27
|
||
* email: zhu.yan@alibaba-inc.com
|
||
* tartget: BottomNavigationBar 的示例
|
||
*/
|
||
|
||
import 'package:flutter/material.dart';
|
||
|
||
/*
|
||
* BottomNavigationBar 默认的实例
|
||
* */
|
||
class BottomNavigationBarFullDefault extends StatefulWidget {
|
||
const BottomNavigationBarFullDefault() : super();
|
||
@override
|
||
State<StatefulWidget> createState() => _BottomNavigationBarFullDefault();
|
||
}
|
||
|
||
/*
|
||
* BottomNavigationBar 默认的实例,有状态
|
||
* */
|
||
class _BottomNavigationBarFullDefault extends State {
|
||
int _currentIndex = 1;
|
||
|
||
void _onItemTapped(int index) {
|
||
setState(() {
|
||
_currentIndex = index;
|
||
});
|
||
}
|
||
|
||
@override
|
||
Widget build(BuildContext context) {
|
||
return BottomNavigationBar(
|
||
type: BottomNavigationBarType.fixed, // BottomNavigationBarType 中定义的类型,有 fixed 和 shifting 两种类型
|
||
iconSize: 24.0, // BottomNavigationBarItem 中 icon 的大小
|
||
currentIndex: _currentIndex, // 当前所高亮的按钮index
|
||
onTap: _onItemTapped, // 点击里面的按钮的回调函数,参数为当前点击的按钮 index
|
||
fixedColor: Colors.deepPurple, // 如果 type 类型为 fixed,则通过 fixedColor 设置选中 item 的颜色
|
||
items: <BottomNavigationBarItem> [
|
||
BottomNavigationBarItem(
|
||
title: new Text("Home"), icon: new Icon(Icons.home)),
|
||
BottomNavigationBarItem(
|
||
title: new Text("List"), icon: new Icon(Icons.list)),
|
||
BottomNavigationBarItem(
|
||
title: new Text("Message"), icon: new Icon(Icons.message)),
|
||
|
||
],
|
||
);
|
||
}
|
||
}
|
||
|
||
/*
|
||
* BottomNavigationBar 默认的实例,无状态
|
||
* */
|
||
class BottomNavigationBarLessDefault extends StatelessWidget {
|
||
final widget;
|
||
final parent;
|
||
|
||
const BottomNavigationBarLessDefault([this.widget, this.parent])
|
||
: super();
|
||
|
||
@override
|
||
Widget build(BuildContext context) {
|
||
return BottomNavigationBar(
|
||
);
|
||
}
|
||
} |